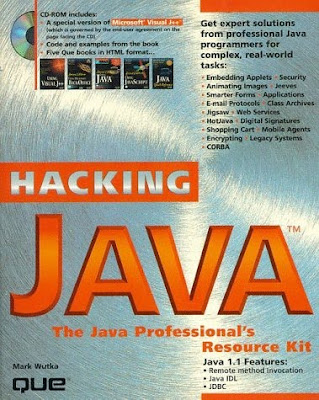
Author: Mark Wutka
Publication Date: Nov-96
ISBN: 1-57521-083-5
Chapter 1: What Is Java?
* Java as a Web Programming Language
* Java as an Applications Programming Language
* New Features on the Horizon
* Java as an Embedded Systems Language
Chapter 2: Embedding Applets in Web Pages
* No Java? No Problem
o Displaying an Image in Place of an Applet
* Passing Parameters to Applets
* Improving Applet Startup Time
Chapter 3: Applet Security Restrictions
* Applet Security
* File Access Restrictions
* Network Restrictions
* Other Security Restrictions
* Getting Around Security Restrictions
o Using Digital Signatures for Increased Access
o Creating a Customized Security Manager
Chapter 4: Displaying Images
* Images in Java
* Displaying Simple Images
o Shrinking and Stretching Images
* Creating Your Own Images
* Displaying Other Image Formats
o The Microsoft Windows Bitmap (BMP) File Format
* Manipulating Images
o Performing Image-Processing Algorithms
* Filtering Image Colors
o Filtering Based on Pixel Position
* Downloading Images
Chapter 5: Animating Images
* Animation
* An Animation Driver
* Animating Image Sequences
* Animating Portions of an Image
* Animating with a Filter
* Cycling the Color Palette
* Animating Graphics
o Redrawing the Entire Screen
o Doing Animation with XOR
* Eliminating Flicker
o Double-Buffering
Chapter 6: Communicating with a Web Server
* Java and Web Servers
* Getting Files Using the URL Class
* Getting Files Using Sockets
* Performing a Query with GET
* Posting Data with the URL Class
* Posting Data Using Sockets
* Supporting the Cookie Protocol
Chapter 7: Creating Smarter Forms
* Smarter Forms
* Creating Forms with the AWT
* Checking for Errors on the Client Side
* Adding Context-Sensitive Help
* Creating Dynamic Forms
* Loading Another URL from an Applet
* Creating Image Maps with Hot Spots
Chapter 8: Reading and Writing Files from an Applet
* Applets and Files
* Using the JFS Filesystem for Applets
o Printing Files Using JFS
o Accessing Other Web Servers from JFS
* Saving Files Using HTTP Post
* Storing and Retrieving Files with FTP
o Sending FTP Commands
o Establishing an FTP Session
o Sending Simple FTP Commands
o Establishing a Data Connection
Chapter 9: Creating Reusable Graphics Components
* Reusable Components
* The Command Pattern
o Invoking Commands from a Menu
* Creating a Reusable Image Button
o Setting the Size of a Canvas
o Handling Input Events
o Painting the Canvas
o Watching for Image Updates
o Creating a CommandImageButton
* Using the Observer Interface
o The Model-View-Controller Paradigm
o Observables and the Model-View-Controller Paradigm
* Using Observables for Other Classes
Chapter 10: Inter-Applet Communication
* Locating Other Applets
* Exchanging Data Using Piped Streams
* Creating Multi-Client Pipes
* Sharing Information with Singleton Objects
Chapter 11: Sending E-Mail from an Applet
* Sending E-Mail
* Sending E-Mail Using the SMTP Protocol
* Accessing Your Mailbox with the POP3 Protocol
Chapter 12: Protecting Applet Code
* Protecting Your Code from Unauthorized Use
* Embedding Copyrights in Your Code
* Verifying the Origin of the Applet
* Hiding Information in Your Applet
* Obfuscating a Working Program
o Make All Your Function and Variable Names Meaningless
o Perform Occasional Useless Computations or Loops
o Hide Small Numbers in Strings
o Create Large Methods
o Spread Methods Out Among Subclasses
o Using a Commercial Obfuscator
Chapter 13: Running Applets as Applications
* Differences Between Applets and Applications
* Allowing an Applet to Run as an Application
* The Applet's Runtime Environment
* Creating an Applet Context
Chapter 14: Creating Your Own Class Archive Files
* Class Archive Files
* Creating Your Own Archive File with Info-ZIP
* Viewing the Contents of a Zip Archive
* Adding Classes Directly to the Browser's Library
* Creating Class Archives with Other Zip Archivers
* Creating Cabinet Files for Internet Explorer
Chapter 15: Accessing Databases with JDBC
* Organizing Your Data for a Relational Database
o Using SQL
o Combining Data from Multiple Tables Using Joins
* Designing Client/Server Database Applications
o Client/Server System Tiers
o Handling Transactions
o Dealing with Cursors
o Replication
o How Does JDBC Work?
o JDBC Security Model
o Accessing ODBC Databases with the JDBC-ODBC Bridge
o JDBC Classes-Overview
o Anatomy of a JDBC Application
o JDBC API Examples
* The Connection Class
* Handling SQL Statements
o Creating and Using Direct SQL Statements
o Creating and Using Compiles SQL Statements (PreparedStatement)
o Calling Stored Procedures (CallableStatement)
* Retrieving Results in JDBC
* Handling Exceptions in JDBC-SQLException Class
* Handling Exceptions in JDBC-SQLWarnings Class
* Handling Date and Time
o java.sql.Date
o java.sql.Time
o java.sql.Timestamp
* Handling SQL Types
o java.sql.Types
* JDBC in Perspective
Chapter 16: Creating 3-Tier Distributed Applications with RMI
* Creating 3-Tier Applications
* RMI Features
* Creating an RMI Server
o Defining a Remote Interface
o Creating the Server Implementation
o Creating the Stub Class
* Creating an RMI Client
* Creating Peer-to-Peer RMI Applications
* Garbage Collection, Remote Objects, and Peer-to-Peer
Chapter 17: Creating CORBA Clients
* Defining IDL Interfaces
* Compiling IDL Interfaces for Java Clients
* Writing a Client Applet
* Handling Exceptions
* CGI Programs, Java.net.*, and Java.io.* May Not Be the Best Choices
* Using the Dynamic Invocation Interface and the Interface Repository
* Using Filters
* Some Points About Distributed System Architecture
Chapter 18: Using CORBA IDL with Java
* What Is CORBA?
* Sun's IDL to Java Mapping
o IDL Modules
o IDL Constants
o IDL Data Types
o Enumerated Types
* Structures
o Unions
o Sequences and Arrays
o Exceptions
o Interfaces
o Attributes
* Using CORBA in Applets
o Choosing Between CORBA and RMI
* Creating CORBA Clients with JavaIDL
* Creating CORBA Clients with VisiBroker
Chapter 19: Creating CORBA Servers
* Creating a Basic CORBA Server
o Using Classes Defined by IDL Structs
o VisiBroker Skeletons
o Using the VisiBroker TIE Interface
o JavaIDL Skeletons
* Creating Callbacks in CORBA
* Wrapping CORBA Around an Existing Object
o Mapping to and from CORBA-Defined Types
o Creating Remote Method Wrappers
o Implementing Wrapped Callbacks
Chapter 20: Increasing Graphics Performance
* Double-Buffering to Speed Up Drawing
o Detecting the Best Drawing Method at Runtime
o Creating an Autodetecting update Method
* Performing Selective Updates
* Redrawing Changed Areas
Chapter 21: Download Strategies
* Huffman Coding and Lempel-Ziv Compression
* Delayed Downloading
o Delayed Instantiation
o Downloading in the Background
* Providing Local Libraries
o Installing Local Libraries for Hotjava and Appletviewer
o Installing Local Libraries for Netscape
o Installing Local Libraries for Internet Explorer
* Downloading Classes in Zipped Format
o Zip Downloading in Netscape Navigator Version 3
o A Zipfile Class Loader
* Packaging Classes in Jars and Cabinets
Chapter 22: Faster Image Downloads
* Reducing Image Size
* Image Strips
o Using the Graphics.clipRect Method
o Creating Another Graphics Context
* Storing Only Parts on an Image Strip
Chapter 23: Creating Web Services in Java
* Using Java Objects Instead of CGI
* The Servlet API
* The Web Server as a Computing Server
* Adding Web Access to Your Java Applications
* Migrating off the Web Server in the Future
Chapter 24: Writing Web Services for Jeeves
* What Is Jeeves?
* The Jeeves HTTP Server
o Architectural Overview
o Installing and Running the Jeeves HTTP Server
o Administering the Jeeves Web Server
o HTTP Server Security
* Extending Jeeves' Functionality with Servlets
o Employing the Servlet API
o Using the Jeeves Development Toolkit
* Building a Database Servlet
o Getting the Information from the Users
o Connecting Your Servlet to a JDBC Database
o Inserting Data in the Database
o Searching the Database
* Building a Simple Autonomous Agent System with Jeeves
o Using Object Serialization to Transport Agents Across the Internet
o Building the Remote Agency
o Creating a Generic Agent Interface
o Implementing a Database Search Agent
o Building the Home Agency
o Launching the Agent
o Debriefing the Agent
Chapter 25: Writing Web Services for Jigsaw
* Architectural Overview
o Handling the HTTP Protocol with the Daemon Module
o Managing the Server Information Space with the Resource Module
o Maintaining Server State via Object Persistence
o Pre and Post Request Processing with Resource Filters
* Jigsaw Interface
o The HTTPResource Class
o The FilteredResource Class
o The DirectoryResource Class
o The FileResource Class
* Installation and Setup of the Jigsaw HTTP Server
* Adding Content to the Jigsaw Server
* Extending the Server with Java
* Writing Resource Filters in Java
* Handling Forms and the POST Method in Java
Chapter 26: Securing Applets with Digital Signatures
* What Are Digital Signatures?
* Allowing More Access for Signed Applets
* Using a Third Party for Applet Signatures
* Potential Security Problems with Digital Signatures
o Using Phony Signatures
o Receiving Old Software
o Mistaken Trust in Signed Applets
o Running a Phony Web Browser
* Obtaining a Digital Signature Certificate
* Other Uses for Digital Signatures
Chapter 27: Encrypting Data
* Choosing the Right Kind of Encryption
* Guarding Against Malicious Attacks
o Resisting a Playback Attack
o Don't Store Keys in Your Applets
o Using Public Key Encryption to Exchange Session Keys
o Using Secure HTTP to Thwart Impersonations
* Getting Encryption Software
o Getting SSLava, the Secure Sockets Library
o Getting the Cryptix Library
o Getting the Acme Crypto Package
Chapter 28: Accessing Remote Systems Securely
* Getting a Secure Web Server
* Preventing Impersonations
* Accessing Remote Data
* Passing Keys to Clients
o Don't Reuse Symmetric Keys
o Using Public Key Encryption to Get a Private Key
o Passing a Private Key as an Applet Parameter
* Implementing a Single-Client Secure Server
* Implementing a Multiclient Secure Server
* Creating Other Secure Remote Access Programs
Chapter 29: Creating a Java Shopping Cart
* Designing a Basic Shopping Cart
* Creating a Shopping Cart User Interface
o Creating a Catalog Applet
o Creating the Shopping Cart Applet
Chapter 30: Performing Secure Transactions
* Letting Customers Digitally Sign Orders
* Using Encryption in All Network Communications
* Creating Java Services for Netscape Servers
o Creating a Server-Side "Hello World"
o Installing a New Server-Side Java Applet
o Handling Forms from Server-Side Applets
o Sending Files as a Response
o Returning Multi-Part Responses
o Maintaining Information Between Applet Invocations
* Making Server-Side Applets Work on Different Web Servers
* Performing Secure Transactions
Chapter 31: Java Electronic Commerce Framework (JECF)
* The Difficulties of Electronic Commerce
o Theft of Information
o Fraudulent Programs
o Proprietary Solutions
o Static Solutions
o Platform-Dependence
* Creating Online Services with the JECF
* Storing Information in the Wallet Database
o Keeping Data Safe
o Performing Transactions
* Implementing a Shopping Cart Applet with the JECF
* Offering Services with Cassettes
o Creating Other Wallet Services
o Ensuring Cassette Security
o Dealing with System Failures
* JECF Availability
* Getting More Information About the JECF
Chapter 32: Encapsulating Legacy Systems
* Focusing on Function, not Form
* Providing Access to New Systems
* Using CORBA to Open Up a Closed System
* Encapsulating a TCP/IP System
* Encapsulating with Native Method Calls
o Wrapping Java Around a Native Interface
o Writing Native Methods in C
* Encapsulating by Emulating a User
* Getting Assistance from the Legacy System
* Presenting a Different Interface
* Combining Multiple Systems
o Handling Deletions Originating in the Legacy System
o Using a Two-Phase Commit Protocol
o Implementing a Two-Phase Commit
* Some Real-World Examples
o An Example Legacy System
o Creating a New Application for the Existing Terminal Base
o Creating a New Interface for an Existing Application
o Clearing a Path for Migration off the Legacy System
Chapter 33: Web-Enabling Legacy Systems
* Using Encapsulations to Access Legacy Data
o Aiming for Session-Less Transactions
o Storing Session Information in the Web Page
o Using HTTP Cookies to Preserve Session Information
o Choosing a Good Session Identifier
o Clearing Out Old Sessions
* Accessing Legacy Data from Servlets
Chapter 34: Interfacing with CICS Systems
* A Thumbnail Sketch of CICS
* The CICS External Call Interface
* The Java-CICS Gateway API
* Creating Multiple-Call LUWs
* Creating Web Interfaces to CICS
* Providing a CORBA Interface to CICS
o Creating a CORBA-CICS Gateway
o Creating CORBA Interfaces to CICS Programs
Chapter 35: Adding Additional Protocols to HotJava
* Writing a Protocol Handler
o Step One: Decide Upon a Package Name
o Step Two: Create the Directories
o Step Three: Set Your CLASSPATH
o Step Four: Implement the Protocol
o Step Five: Create the Handler Class
o Step Six: Compile the Sources
* Using Protocol Handlers with HotJava
o Step One: Update the properties File
o Step Two: Run HotJava
* Using Protocol Handlers with Your Own Applications
o The main() Method: Starting FetchWhois
o The FetchWhois Constructor: Where the Work Gets Done
o The whoisUSHFactory Class: Registering the Protocol Handler
o Running FetchWhois
* More on URLStreamHandlerFactory
Chapter 36: Adding New MIME Types to HotJava
* Writing Content Handlers
o Step One: Decide upon a Package Name
o Step Two: Create the Directories
o Step Three: Set Your CLASSPATH
o Step Four: Write the Content Handler
o Step Five: Compile the Source
* Using Content Handlers with HotJava
o Step One: Disable Special MIME Handling
o Step Two: Update the PROPERTIES File
o Step Three: Run HotJava
* Using Content Handlers with Your Own Applications
o Start FetchFuddify
o The ContentHandlerFactory Implementation
o Running the Application
Chapter 37: Creating Multi-User Programs in Java
* Designing Multi-User Applications
* Adding Socket-Based Access to Multi-User Applications
o Creating a Socket-Based Server
o Sending Messages over Sockets
* Other Issues When Dealing with Sockets
* Adding RMI Access to Multi-User Applications
Chapter 38: Creating On-Demand Multimedia Services
* Java's Suitability for On-Demand Applications
* Using the On-Demand Audio Applet
o Logging In
o Playing Audio Clips
* Adding Sound to Applets
* On-Demand Music Applet Code Review
o Applet Architecture
o Initialization and Registration
o Song Selection
o Playing the Songs
* Java Shortcomings
* New Features
Chapter 39: Implementing a Multimedia Encyclopedia
* Java's Suitability for Multimedia Applications
o Java Is Portable
o Java Is Compact
o Java Can Handle Streaming Data
o Java Is Based on the Client/Server Model
o Java Supports PDAs Easily
* Using the Multimedia Encyclopedia
* Adding Images and Sound to Applets
* The On-Line Multimedia Encyclopedia In-Depth
o Applet Architecture
o Index Window
o Topic Window
* Shortcomings
* New Features
Chapter 40: Implementing Java Interfaces for Non-Traditional Devices
* Characteristics of Non-Traditional Devices
* The New Computing Model
* Designing Applications to Support Non-Traditional Devices
o Separating the User Interface from the Application
o Avoiding Large, Monolithic Applications
o Sticking to Standard Libraries
o Avoiding Long, Complex Transactions
* Designing User Interfaces for Small Devices
o Creating Obvious, Self-Documenting Interfaces
o Avoiding Extraneous Pictures or Information
o Keeping Everything Readable
o Supporting Multiple Sources of Input
* Creating Reusable Components for Small Devices
o Using the CardLayout Layout Manager as a Stack
o Creating a Keyboard/Keypad Input Filter
o Creating a Pop-Up Keypad for Pen and Touch-Screen Users
Another Hacker Books
Another Java Books
Download
No comments:
Post a Comment