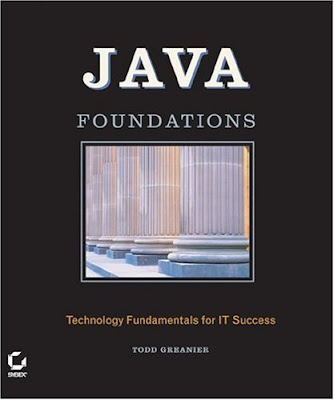
Contents
Introduction xiii
Chapter 1 The History of Java 1
Where Java Technology Came From . . . . . . . . . . . . . . . . . 2
The Green Project . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
Enter the Web . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
The Features of Java Technology . . . . . . . . . . . . . . . . . . . . 3
Java Is Simple . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Java Is Object Oriented . . . . . . . . . . . . . . . . . . . . . . . . . 4
Java Is Interpreted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Java Is Portable . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Java Is Robust . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Java Is Secure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
Java Is Multithreaded . . . . . . . . . . . . . . . . . . . . . . . . . . 8
Java Is High Performance . . . . . . . . . . . . . . . . . . . . . . . 9
Java Saves Time and Money . . . . . . . . . . . . . . . . . . . . . 9
Java Solves Impotant Problems . . . . . . . . . . . . . . . . . 10
How Java Compares with Other Languages . . . . . . . . . . 10
How to Download and Install Java . . . . . . . . . . . . . . . . . 11
Downloading the J2SE Software . . . . . . . . . . . . . . . . . 12
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
Chapter 2 Java Fundamentals 23
Creating a Java Program . . . . . . . . . . . . . . . . . . . . . . . . . 24
The HelloWorld Program . . . . . . . . . . . . . . . . . . . . . . . . 24
Writing the HelloWorld Source Code . . . . . . . . . . . . . 25
Compiling the HelloWorld Source Code . . . . . . . . . . . 26
Executing the HelloWorld Program . . . . . . . . . . . . . . . 27
Examining the Source Code . . . . . . . . . . . . . . . . . . . . . . . 28
Using Comments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
Using White Space . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
Defining the Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
Defining the Method . . . . . . . . . . . . . . . . . . . . . . . . . . 34
Wrapping Up the HelloWorld Program . . . . . . . . . . . . 38
Working with Arguments in the
main()
Method . . . . . 39
The Basic Java Data Types . . . . . . . . . . . . . . . . . . . . . . . . 41
Literal Values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42
The Integer Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43
The Floating Point Types . . . . . . . . . . . . . . . . . . . . . . . 45
The Character Type . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
The Boolean Type . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
Using the Primitive Types . . . . . . . . . . . . . . . . . . . . . . 46
The
String
Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
Primitive Values versus Reference Values . . . . . . . . . . . 47
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52
Chapter 3 Keywords and Operators 53
Creating Valid Names in Java . . . . . . . . . . . . . . . . . . . . . 54
The Keyword List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
The Primitive Type Keywords . . . . . . . . . . . . . . . . . . . 56
The Flow Control Keywords . . . . . . . . . . . . . . . . . . . . 56
Modification Keywords . . . . . . . . . . . . . . . . . . . . . . . . 57
Class-Related Keywords . . . . . . . . . . . . . . . . . . . . . . . 60
Object-Related Keywords . . . . . . . . . . . . . . . . . . . . . . 69
Wrapping Up the Keywords . . . . . . . . . . . . . . . . . . . . . 74
The Java Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 74
The Arithmetic Operators . . . . . . . . . . . . . . . . . . . . . . 75
The Assignment Operators . . . . . . . . . . . . . . . . . . . . . 80
The Relational Operators . . . . . . . . . . . . . . . . . . . . . . 81
The Conditional Operators . . . . . . . . . . . . . . . . . . . . . 83
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 86
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87
Chapter 4 Flow Control 89
Application Scope . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
The if Statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
Adding the else Statement . . . . . . . . . . . . . . . . . . . . . . 92
Testing the Array of Arguments . . . . . . . . . . . . . . . . . . 94
The switch and case Statements . . . . . . . . . . . . . . . . . . . . 97
The default Statement . . . . . . . . . . . . . . . . . . . . . . . . 100
Deciding between if/else and switch/case . . . . . . . . . . 100
Processing a Range of Values . . . . . . . . . . . . . . . . . . . 102
The Ternary Operator . . . . . . . . . . . . . . . . . . . . . . . . . . 104
The for Loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105
Multiple Increment Steps . . . . . . . . . . . . . . . . . . . . . . 107
Beware the Infinite Loop . . . . . . . . . . . . . . . . . . . . . . 109
The while Loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 110
Comparing for and while Loops . . . . . . . . . . . . . . . . 111
The do Statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113
The Branching Statements . . . . . . . . . . . . . . . . . . . . . . . 114
The break Statement . . . . . . . . . . . . . . . . . . . . . . . . . 114
The continue Statement . . . . . . . . . . . . . . . . . . . . . . . 117
The return Statement . . . . . . . . . . . . . . . . . . . . . . . . . 121
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124
Chapter 5 Arrays 125
Understanding Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . 126
Declaring Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 128
Creating Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129
Getting the Length of an Array . . . . . . . . . . . . . . . . . 130
Populating an Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
Using Array Initializers . . . . . . . . . . . . . . . . . . . . . . . 133
An Array Initializer Variation . . . . . . . . . . . . . . . . . . 134
Accessing Array Elements . . . . . . . . . . . . . . . . . . . . . . . 135
Multidimensional Arrays . . . . . . . . . . . . . . . . . . . . . . . . 137
Two-Dimensional Array Initializers . . . . . . . . . . . . . . 140
Nonrectangular Arrays . . . . . . . . . . . . . . . . . . . . . . . 141
The java.util.Arrays Class . . . . . . . . . . . . . . . . . . . . . . . 142
Filling an Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 142
Sorting an Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
Searching an Array . . . . . . . . . . . . . . . . . . . . . . . . . . 144
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 146
Chapter 6 Introduction to Object-Oriented Programming 147
The Object-Oriented Paradigm . . . . . . . . . . . . . . . . . . . 148
Real-World Objects . . . . . . . . . . . . . . . . . . . . . . . . . . 149
Defining a Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 150
Instantiating and Using Objects . . . . . . . . . . . . . . . . . . . 152
A Closer Look at a Lamp Object . . . . . . . . . . . . . . . . 153
Sharing a Reference . . . . . . . . . . . . . . . . . . . . . . . . . . 155
Object Messaging: Adding a Lightbulb . . . . . . . . . . . . . 156
Passing by Value . . . . . . . . . . . . . . . . . . . . . . . . . . . . 160
Passing by Reference . . . . . . . . . . . . . . . . . . . . . . . . . 162
The this Keyword . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
Bypassing Local Variables Using this . . . . . . . . . . . . . 166
Passing a Reference Using this . . . . . . . . . . . . . . . . . . 168
Static Methods Have No this Reference . . . . . . . . . . 169
Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
Multiple Constructors . . . . . . . . . . . . . . . . . . . . . . . . 173
Constructor Chaining . . . . . . . . . . . . . . . . . . . . . . . . 177
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 180
Chapter 7 Advanced Object-Oriented Programming 181
Claiming Your Inheritance . . . . . . . . . . . . . . . . . . . . . . . 182
Using the extends Keyword . . . . . . . . . . . . . . . . . . . . . . 184
The Rules of Inheritance . . . . . . . . . . . . . . . . . . . . . . 185
Reference Types versus Runtime Types . . . . . . . . . . . 187
Expanding the Subclasses . . . . . . . . . . . . . . . . . . . . . . 190
The Class Hierarchy . . . . . . . . . . . . . . . . . . . . . . . . . 193
The Reference Type Rule for Methods . . . . . . . . . . . . 195
The instanceof Operator and Object Casting . . . . . . . . . 196
Object Casting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197
Introducing Polymorphism . . . . . . . . . . . . . . . . . . . . . . . 199
Method Overloading . . . . . . . . . . . . . . . . . . . . . . . . . 199
Method Overriding . . . . . . . . . . . . . . . . . . . . . . . . . . 201
Abstract Classes and Methods . . . . . . . . . . . . . . . . . . . . 212
Interfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 215
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 219
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 220
Chapter 8 Exception Handling 221
The Method Call Stack . . . . . . . . . . . . . . . . . . . . . . . . . . 222
Exception Noted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 223
The Exception Hierarchy . . . . . . . . . . . . . . . . . . . . . . 224
Handling Those Exceptions . . . . . . . . . . . . . . . . . . . . . . 226
Using try and catch . . . . . . . . . . . . . . . . . . . . . . . . . . 227
Using a finally Clause . . . . . . . . . . . . . . . . . . . . . . . . 232
Creating Your Own Exception Type . . . . . . . . . . . . . . . 235
Throwing Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . 237
Using the throws Keyword . . . . . . . . . . . . . . . . . . . . . 238
The throw Keyword . . . . . . . . . . . . . . . . . . . . . . . . . . 240
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247
Chapter 9 Common Java API Classes 249
The java.lang.String Class . . . . . . . . . . . . . . . . . . . . . . . 250
Common String Methods . . . . . . . . . . . . . . . . . . . . . . 251
The java.lang.StringBuffer Class . . . . . . . . . . . . . . . . . . . 260
The java.lang.Math Class . . . . . . . . . . . . . . . . . . . . . . . . 264
Calculating a Random Number . . . . . . . . . . . . . . . . . 266
The Wrapper Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . 270
Creating Wrapper Objects . . . . . . . . . . . . . . . . . . . . . 271
Common Wrapper Methods . . . . . . . . . . . . . . . . . . . 273
The Character Class . . . . . . . . . . . . . . . . . . . . . . . . . 276
Wrapping It Up . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 278
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 278
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 279
Chapter 10 The Collections Framework 281
Defining a Framework . . . . . . . . . . . . . . . . . . . . . . . . . . 282
The java.util.Collection Interface . . . . . . . . . . . . . . . . . . 282
Understanding Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . 284
The java.util.List Interface . . . . . . . . . . . . . . . . . . . . . 284
The
java.util.ArrayList
Class . . . . . . . . . . . . . . . . . . . 285
Summarizing Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . 291
Understanding Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 291
The java.util.Set Interface . . . . . . . . . . . . . . . . . . . . . 291
The java.util.HashSet Class . . . . . . . . . . . . . . . . . . . . 292
Summarizing Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . 294
Understanding Maps . . . . . . . . . . . . . . . . . . . . . . . . . . . 294
The java.util.Map Interface . . . . . . . . . . . . . . . . . . . . 294
The java.util.HashMap Class . . . . . . . . . . . . . . . . . . . 296
Summarizing Maps . . . . . . . . . . . . . . . . . . . . . . . . . . 298
Working with Iterators . . . . . . . . . . . . . . . . . . . . . . . . . 298
The java.util.Iterator Interface . . . . . . . . . . . . . . . . . . 299
The java.util.ListIterator Interface . . . . . . . . . . . . . . . 301
Iterators and Maps . . . . . . . . . . . . . . . . . . . . . . . . . . 304
Terms to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 306
Review Questions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 307
Appendix A Answers to Review Questions 309
Chapter 1 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 309
Chapter 2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 310
Chapter 3 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 310
Chapter 4 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 311
Chapter 5 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 313
Chapter 6 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 314
Chapter 7 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 315
Chapter 8 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 316
Chapter 9 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 317
Chapter 10 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 317
Glossary 319
Index 329
Another Java Books
Download
No comments:
Post a Comment