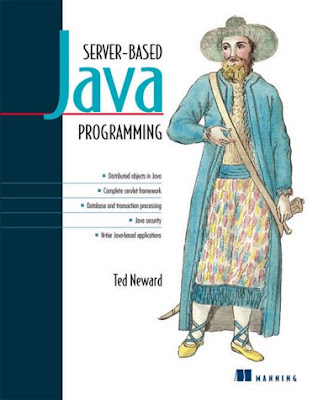
contents
foreword xv
preface xvii
acknowledgments xxi
about this book xxiii
goals of this book xxix
about the author xxxi
about the cover illustration xxxiii
1 Enterprise Java 1
1.1 Enterprise development 1
What is enterprise development? 1 G Developing the enterprise
application 4 G Reinventing the wheel 7
1.2 Three zeroes 8
Zero development 9 G Zero deployment 11 G Zero administration 12
1.3 Java in the enterprise 14
Sun’s view 14 G Alternate views 14
1.4 Why Java? 15
Criticisms of Java as a server-side language 17
1.5 Summary 25
1.6 Additional reading 26
2 ClassLoaders 27
2.1 Dynamic linking 28
Run-time dynamic loading 28 G Reflection 31
2.2 ClassLoaders: rules and expectations 37
Java .class file format 37 G Using ClassLoader 39
java.lang.ClassLoader 41 G Java name spaces 48
2.3 Java’s built-in ClassLoaders 49
java.security.SecureClassLoader 49 G java.net.URLClassLoader 49
sun.applet.AppletClassLoader 57 G java.rmi.server.RMIClassLoader 57
Bootstrap ClassLoader 57 G sun.misc.Launcher$ExtClassLoader 58
2.4 Summary 58
2.5 Additional reading 59
3 Custom ClassLoaders 61
3.1 Extending ClassLoader 61
FileSystemClassLoader 62 G HashtableClassLoader 66
CompilerClassLoader 67 G StrategyClassLoader and
ClassLoaderStrategy 71 G CompositeClassLoader 75
Other ClassLoader tricks 79 G Other ClassLoaders 80
3.2 On-the-fly code upgrades 80
3.3 GJAS: first steps 85
Goals 85 G Service 86 G Server 88 G ServerManager 90
3.4 Summary 92
4 Extensions 93
4.1 Types of extensions 94
Installed extensions 94 G Building an installed extension 95
Download extensions 96 G Building a download extension 98
4.2 Implications of the extensions mechanism 100
Distributed libraries through download extensions 100
Java EXEs; relation to C++ static linking 101
4.3 Packaging extensions 102
The build-time vs. run-time dilemma 103
4.4 The plug-in 104
The plug-in concept 105 G Enter plug-ins 107 G Marking a .jar file as
a plug-in 110 G PluginClassLoader 111 G Example:
PluginApp 118 G Uses for plug-ins 124
4.5 Summary 125
5 Threads 126
5.1 Why threads? 127
Concurrent processing 127 G Scalability per machine 128
Encapsulation 129 G Design and implementation 130
5.2 Java threads 130
java.lang.Thread and java.lang.Runnable 131 G Starting threads 137
Stopping threads 139 G Daemon threads 142 G Threads and
ClassLoaders 143 G java.lang.ThreadGroup 144
5.3 Thread implementations in Java 146
Green threads 147 G Native threads 147 G Hybrids 147
Implications 148
5.4 Summary 148
5.5 Additional reading 148
6 Threading issues 149
6.1 Synchronization 150
Thread-local storage 152
6.2 Exception-handling with multiple threads 153
6.3 Thread idioms and patterns 158
Client-Dispatcher-Server 158 G Fire-and-forget 159
Active Object 160 G SpinLoop 160
Polling (PeriodicThread) 161 G DelayedFire
(ScheduledThread) 163 G Futures 164
6.4 GJAS 166
Adding thread support to GJAS 167
6.5 Summary 173
6.6 Additional reading 173
7 Control 174
7.1 GJAS 175
Local implementation 175 G Example: HelloService 186
7.2 Testing the LocalServer implementation 187
7.3 ExecService 189
7.4 HelloAgainService 193
ThreadServer 196 G Example: ConsoleControlService 201
8 Remote control 208
8.1 RMI implementation 209
Analysis 217
8.2 Other implementations 218
8.3 Necessary improvements 219
8.4 Additional reading 224
9 Configuration 225
9.1 Java models 225
Interface: ConfigProperty and ConfigProperties 226 G Usage 233
Configuration front ends 235
9.2 Summary 236
10 Sockets 237
10.1 Simple socket services 237
SocketClient 238 G EchoService 243 G TimeService 245
Analysis 246
10.2 Encapsulation and refactoring 247
SocketServer 247 G Example: Echo2Service 254
10.3 Connection and ConnectionManager 255
Example: EchoConnection 262 G Example: HTTPConnection 263
Servlets 272
10.4 Advanced Socket services 273
SocketClassLoader and SocketClassService 273
Concept: RedirectorService 279 G Concept: FilterService 280
Other types 281
10.5 Summary 281
10.6 Additional reading 282
11 Servlets 283
11.1 Relationship to sockets 283
CodeServlet: A filtering servlet 285 G HeaderFooter: a redirecting
servlet 287 G Server-side scripting capabilities 289 G Servlets: Not just
about HTML anymore 290
11.2 Servlets and the n-tier application 292
Separating logic from content 293
11.3 Servlets as a poor man’s RMI 293
Example: RemoteStorageServlet 295 G Concept: poor man’s RMI 297
Concept: SOAP 298
11.4 Summary 298
11.5 Additional reading 298
12 Persistence 300
12.1 Java Serialization 301
Serialization to other places 302 G Security and Serialization 303
Customized Serialization 306 G Serialization and evolution 309
Replacement 313
12.2 Beyond the specification 317
Remote storage of objects 317 G Example: RemoteStorageService and
RemoteStorageClient 318 G Remote construction of objects 323
Example: RemoteObjectFactory 325
12.3 JDBC 330
Transient data, state data, data that Isn’t data 332
Example: JDBCClassLoader 334
12.4 Summary 338
12.5 Additional reading 339
13 Business objects 340
13.1 Modeling data 340
Two-tier Systems vs. n-tier Systems 341 G One-tier systems 341
Two-tier systems 342 G n-tier systems 342 G Benefits of an n-tier
model 343 G Business objects, entity relationships 346 G Example:
employee directory 346 G Business objects layer interface layer 348
13.2 Using the Business Object layer 366
Classic Presentation Code: GUIs 366 G Example: OrgTree 366
Feeling cheated? 370
13.3 Summary 370
13.4 Additional reading 371
14 Business object models 372
14.1 Example: HashtableModel 372
Overview 373 G HashtablePerson, HashtableEmployee,
HashtableManager 374 G HashtableModel: Creating objects 375
HashtableModel: Finding objects 378 G HashtableModel: Removing
objects 379 G Conclusion 379
14.2 Example: RDBMSModel 380
RDBMSModel: Storing Business Objects in an RDBMS 381
Overview 382 G RDBMSPerson, RDBMSEmployee,
RDBMSManager 384 G RDBMSModel: Creating objects 391
RDBMSModel: Finding objects 394 G RDBMSModel: Removing
objects 395 G Conclusion 397
14.3 Summary 400
14.4 Additional reading 401
15 Middleware 402
15.1 Why distribute? 402
Communication 403 G Performance 404 G Economics (clustering/
fault-tolerance) 405 G Reliability (clustering/load-balancing) 406
15.2 Distributed object design vs. classic object design 406
Stateful vs. stateless 406
15.3 Technologies 410
Raw access: Sockets 411 G Java RPC: remote method invocation 412
Analysis 417 G RMI/JRMP 419 G Object Request Brokers:
CORBA 428 G Object Request Brokers: Distributed Component Object
Model 432 G Message-Oriented Middleware: JMS 433
Objects across the wire: Mobile objects 435 G Objects across
the wire: shared objects 441
15.4 Employee middleware models 448
RMI implementation 451 G JSDTModel: Shared-object
implementation 452 G Analysis 460
15.5 Additional reading 461
16 Java Native Interface 463
16.1 Java Native Interface 464
Native code on the server 465
16.2 JNI essentials 472
Java calling native 472 G Native calling Java 478
JNI invocation 484 G JNI changes in JDK 1.2 492
16.3 Other methods of Java-to-native interaction 494
Sockets 494 G CORBA 494
16.4 Integrating the server: GJAS goes native 495
Making GJAS an NT service 495 G Using NT IPC mechanisms:
Named pipe 496
16.5 Other JNI uses 506
Debugging support 506 G JVMDI 507 G JVMPI 508
16.6 Summary 508
16.7 Additional reading 508
17 Monitoring 510
17.1 Impotance grows 510
Liveness 511 G Notification 523
17.2 Summary 533
epilogue 535
index 547
Download
Another Web Programming Books
No comments:
Post a Comment