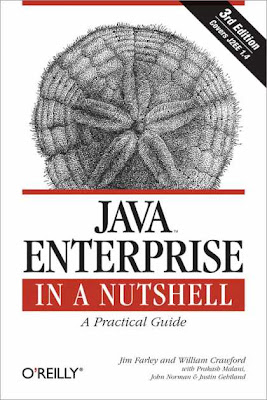
Table of Contents
Preface.................................................................................................................................................................1
0.1. Contents of This Book......................................................................................................................1
0.2. Related Books..................................................................................................................................2
0.3. Java Programming Resources Online...............................................................................................2
0.4. Examples Online..............................................................................................................................3
0.5. Conventions Used in This Book.......................................................................................................4
0.6. We'd Like to Hear from You............................................................................................................5
0.7. Acknowledgments............................................................................................................................6
0.7.1. David Flanagan.................................................................................................................6
0.7.2. Jim Farley.........................................................................................................................6
0.7.3. William Crawford.............................................................................................................6
0.7.4. Kris Magnusson................................................................................................................6
1.1. Enterprise Computing Defined.........................................................................................................8
1.2. Enterprise Computing Demystified..................................................................................................8
1.3. The Java Enterprise APIs..................................................................................................................9
1.3.1. JDBC: Working with Databases.....................................................................................10
1.3.2. RMI: Remote Method Invocation...................................................................................10
1.3.3. Java IDL: CORBA Distributed Objects..........................................................................11
1.3.4. JNDI: Accessing Naming and Directory Services..........................................................11
1.3.5. Enterprise JavaBeans......................................................................................................12
1.3.6. Servlets...........................................................................................................................12
1.3.7. JMS: Enterprise Messaging............................................................................................13
1.3.8. JTA: Managing Distributed Transactions.......................................................................13
1.4.1. Enabling E−Commerce for a Mail−Order Enterprise.....................................................14
1.4.2. Updating CornCo with Enterprise JavaBeans................................................................15
1.4. Enterprise Computing Scenarios.....................................................................................................16
1.5. Java Enterprise APIs Versus Jini....................................................................................................17
2.1. JDBC Architecture.........................................................................................................................18
2.2. JDBC Basics..................................................................................................................................20
2.3. JDBC Drivers.................................................................................................................................20
2.3.1. JDBC URLs....................................................................................................................21
2.3.2. The JDBC−ODBC Bridge..............................................................................................23
2.4. Connecting to the Database............................................................................................................24
2.5. Statements......................................................................................................................................24
2.5.1. Multiple Result Sets........................................................................................................25
2.6.1. Handling Nulls................................................................................................................26
2.6.2. Large Data Types............................................................................................................27
2.6.3. Dates and Times..............................................................................................................28
2.6. Results............................................................................................................................................29
2.7. Handling Errors..............................................................................................................................30
2.7.1. SQL Warnings................................................................................................................31
2.8. Prepared Statements.......................................................................................................................31
2.9. Metadata.........................................................................................................................................32
2.9.1. DatabaseMetaData..........................................................................................................33
2.9.2. ResultSetMetaData.........................................................................................................34
2.10. Transactions.................................................................................................................................34
2.11. Stored Procedures........................................................................................................................36
2.12. Escape Sequences........................................................................................................................38
2.13. JDBC 2.0......................................................................................................................................40
2.13.1. Results Handling...........................................................................................................41
2.13.2. Batch Updates...............................................................................................................43
2.13.3. Java−Aware Databases.................................................................................................43
2.13.4. BLOBs and CLOBs......................................................................................................45
2.13.5. The JDBC Standard Extension.....................................................................................46
3.1. Introduction to RMI........................................................................................................................47
3.1.1. RMI in Action.................................................................................................................47
3.1.2. RMI Architecture............................................................................................................49
3.1.3. RMI Object Services.......................................................................................................49
3.1.3.1. Naming/registry service...............................................................................................50
3.1.3.2. Object activation service..............................................................................................53
3.1.3.3. Distributed garbage collection.....................................................................................55
3.2.1. Key RMI Classes for Remote Object Implementations..................................................55
3.2. Defining Remote Objects................................................................................................................55
3.3. Creating the Stubs and Skeletons....................................................................................................56
3.4. Accessing Remote Objects as a Client............................................................................................56
3.4.1. The Registry and Naming Services................................................................................59
3.4.2. Remote Method Arguments and Return Values.............................................................60
3.4.3. Factory Classes...............................................................................................................61
3.5. Dynamically Loaded Classes..........................................................................................................61
3.5.1. Configuring Clients and Servers for Remote Class Loading..........................................63
3.5.2. Loading Classes from Applets........................................................................................64
3.6. Remote Object Activation...............................................................................................................65
3.6.1. Persistent Remote References.........................................................................................66
3.6.2. Defining an Activatable Remote Object.........................................................................68
3.6.2.1. The Activatable class...................................................................................................68
3.6.2.2. Implementing an activatable object.............................................................................70
3.6.3. Registering Activatable Objects.....................................................................................70
3.6.3.1. Registering an activatable object without instantiating...............................................70
3.6.3.2. Passing data with the MarshalledObject......................................................................71
3.6.4. Activation Groups...........................................................................................................73
3.6.4.1. Registering activation groups......................................................................................74
3.6.4.2. Assigning activatable objects to groups.......................................................................75
3.6.5. The Activation Daemon..................................................................................................75
3.6.5.1. The daemon's dual personality.....................................................................................76
3.7.1. RMI with JNI Versus CORBA.......................................................................................76
3.7. RMI and Native Method Calls........................................................................................................77
3.8. RMI over IIOP...............................................................................................................................78
3.8.1. Accessing RMI Objects from CORBA...........................................................................78
4.1. The CORBA Architecture...............................................................................................................81
4.1.1. Interface Definition Language........................................................................................81
4.1.2. Object Request Broker....................................................................................................84
4.1.3. The Naming Service.......................................................................................................85
4.1.4. Inter−ORB Communication............................................................................................85
4.2. Creating CORBA Objects...............................................................................................................86
4.2.1. An IDL Primer................................................................................................................86
4.2.1.1. Modules.......................................................................................................................87
4.2.1.2. Interfaces.....................................................................................................................88
4.2.1.3. Data members and methods.........................................................................................89
4.2.1.4. A complete IDL example.............................................................................................89
4.2.2. Turning IDL Into Java....................................................................................................90
4.2.2.1. A simple server class...................................................................................................90
4.2.2.2. The helper class...........................................................................................................90
4.2.2.3. The holder class...........................................................................................................91
4.2.2.4. The client and server stubs...........................................................................................91
4.2.3. Writing the Implementation............................................................................................92
4.3. Putting It in the Public Eye.............................................................................................................93
4.3.1. Initializing the ORB........................................................................................................95
4.3.2. Registering with a Naming Service................................................................................96
4.3.3. Adding Objects to a Naming Context.............................................................................98
4.4. Finding Remote Objects.................................................................................................................99
4.4.1. Initial ORB References.................................................................................................100
4.4.2. Getting Objects from Other Remote Objects................................................................101
4.4.2.1. Using a naming context.............................................................................................102
4.4.2.2. Using multiple naming services.................................................................................104
4.4.3. Stringified Object References.......................................................................................106
4.5. What If I Don't Know the Interface?............................................................................................106
4.5.1. Dynamic Invocation Interface......................................................................................106
5.1. The Servlet Life Cycle..................................................................................................................107
5.2. Servlet Basics...............................................................................................................................109
5.2.1. HTTP Servlets...............................................................................................................111
5.2.2. Forms and Interaction...................................................................................................112
5.2.3. POST, HEAD, and Other Requests..............................................................................115
5.2.4. Servlet Responses.........................................................................................................115
5.2.5. Servlet Requests............................................................................................................117
5.2.6. Error Handling..............................................................................................................118
5.2.6.1. Status codes................................................................................................................119
5.2.6.2. Servlet exceptions......................................................................................................120
5.2.6.3. A file serving servlet..................................................................................................121
5.2.7. Security........................................................................................................................121
5.8.1. HttpSessionBindingListener.........................................................................................122
5.8.2. Session Contexts...........................................................................................................123
5.3. Servlet Chaining..........................................................................................................................124
5.4. Custom Servlet Initialization........................................................................................................124
5.5. Thread Safety...............................................................................................................................125
5.6. Server−Side Includes....................................................................................................................126
5.7. Cookies........................................................................................................................................127
5.8. Session Tracking..........................................................................................................................130
5.9. Databases and Non−HTML Content............................................................................................132
5.10. The Servlet API 2.1.....................................................................................................................134
5.10.1. Request Dispatching...................................................................................................136
5.10.2. Shared Attributes........................................................................................................138
5.10.3. Resource Abstraction..................................................................................................138
6.1. JNDI Architecture........................................................................................................................139
6.2. A JNDI Example..........................................................................................................................141
6.3. Introducing the Context................................................................................................................141
6.3.1. Using the InitialContext Class......................................................................................142
6.3.2. Other Naming Systems.................................................................................................142
6.5.1. The Command Interface...............................................................................................144
6.5.2. Loading an Initial Context............................................................................................144
6.5.3. Running the Shell..........................................................................................................146
6.6.1. How Names Work........................................................................................................147
6.6.2. Browsing a Naming System.........................................................................................148
6.6.3. Listing the Bindings of a Context.................................................................................148
6.4. Looking Up Objects in a Context.................................................................................................149
6.5. The NamingShell Application......................................................................................................150
6.6. Listing the Children of a Context.................................................................................................153
6.7. Creating and Destroying Contexts................................................................................................153
6.8. Binding Objects...........................................................................................................................154
6.9. Accessing Directory Services.......................................................................................................155
6.9.1. X.500 Directories..........................................................................................................157
6.9.2. The DirContext Interface..............................................................................................158
6.9.3. The Attributes Interface................................................................................................159
6.9.4. The Attribute Interface..................................................................................................160
6.10. Modifying Directory Entries.......................................................................................................162
6.11. Creating Directory Entries..........................................................................................................163
6.12. Searching a Directory.................................................................................................................163
6.12.1. Search Criteria............................................................................................................164
6.12.2. Search Results.............................................................................................................164
6.12.3. Search Controls...........................................................................................................166
6.12.4. A Search Command....................................................................................................168
7.2.1. The EJB Client..............................................................................................................169
7.2.2. The Enterprise JavaBeans Object.................................................................................171
7.2.3. The EJB Container........................................................................................................171
7.1. A Note on Evolving Standards.....................................................................................................172
7.2. EJB Roles.....................................................................................................................................172
7.3. Transaction Management..............................................................................................................173
7.3.1. Making the EJB Server Aware of Database Transactions............................................175
7.3.2. Transaction Isolation Levels.........................................................................................176
7.4.1. Home Interface.............................................................................................................176
7.4.2. Remote Interface...........................................................................................................177
7.4.3. The Bean Implementation.............................................................................................178
7.4. Implementing a Basic EJB Object................................................................................................180
7.5. Implementing Session Beans........................................................................................................182
7.5.1. Stateless Versus Stateful Session Beans.......................................................................184
7.5.2. Optional Transaction Support.......................................................................................185
7.6. Implementing Entity Beans..........................................................................................................186
7.6.1. Primary Keys................................................................................................................187
7.6.2. Finder Methods.............................................................................................................188
7.6.3. Entity Bean Implementation.........................................................................................188
7.6.3.1. A persistent ProfileBean............................................................................................191
7.6.4. The Entity Context........................................................................................................192
7.6.5. Life Cycle of an Entity Bean........................................................................................194
7.6.6. Handles on Entity Beans...............................................................................................195
7.6.7. Container−Managed Persistence..................................................................................196
7.6.7.1. Handling complex data structures..............................................................................197
7.7. Deploying an Enterprise JavaBeans Object..................................................................................198
7.7.1. Container−Managed Data Mapping.............................................................................199
7.7.2. Access−Control Deployment Attributes.......................................................................204
7.7.3. Generating the Container Classes and Deployment Descriptor....................................204
7.7.4. Packaging Enterprise JavaBeans..................................................................................205
7.8. Using an Enterprise JavaBeans Object.........................................................................................205
7.8.1. Finding Home Interfaces Through JNDI......................................................................209
7.8.2. Creating/Finding Beans................................................................................................210
7.8.3. Using Client−Side Transactions...................................................................................212
7.9. Changes in EJB 1.1 Specification.................................................................................................212
7.9.1. XML−Based Deployment Descriptors.........................................................................213
7.9.2. Entity Beans Required..................................................................................................214
7.9.3. Home Handles...............................................................................................................215
7.9.4. Detailed Programming Restrictions for Bean Implementations...................................215
7.9.5. Assorted Other Changes...............................................................................................216
8.1. Relational Databases.....................................................................................................................217
8.2. Data Types...................................................................................................................................218
8.3. Schema Manipulation Commands................................................................................................219
8.3.1. CREATE TABLE.........................................................................................................219
8.3.2. ALTER TABLE............................................................................................................219
8.3.3. DROP...........................................................................................................................219
8.4. Data Manipulation Commands.....................................................................................................219
8.4.1. SELECT........................................................................................................................221
8.4.1.1. String comparisons....................................................................................................221
8.4.1.2. Subqueries and joins..................................................................................................223
8.4.1.3. Groups........................................................................................................................224
8.4.2. INSERT........................................................................................................................224
8.4.3. UPDATE.......................................................................................................................225
8.4.4. DELETE.......................................................................................................................226
8.5. Functions......................................................................................................................................227
8.5.1. Aggregate Functions.....................................................................................................227
8.5.2. Value Functions............................................................................................................228
8.5.2.1. Date/time functions....................................................................................................229
8.5.2.2. String manipulation functions....................................................................................230
8.6. Return Codes................................................................................................................................231
10.1. IDL Keywords...........................................................................................................................231
10.2. Identifiers...................................................................................................................................232
10.2.1. Mapping Identifiers to Java........................................................................................233
10.3. Comments..................................................................................................................................233
10.3.1. Mapping Comments to Java.......................................................................................233
10.4.1. Strings and Characters................................................................................................233
10.4.1.1. Mapping strings and characters to Java...................................................................234
10.4. Basic Data Types........................................................................................................................235
10.5. Constants and Literals.................................................................................................................238
10.5.1. Mapping Constants to Java.........................................................................................243
10.5.2. Boolean Literals..........................................................................................................244
10.5.3. Numeric Literals.........................................................................................................245
10.5.3.1. Integer literals..........................................................................................................245
10.5.3.2. Floating−point literals..............................................................................................246
10.5.3.3. Fixed−point literals..................................................................................................246
10.5.3.4. Mapping numeric literals to Java.............................................................................247
10.5.4. Character Literals........................................................................................................247
10.5.5. String Literals.............................................................................................................248
10.6. Naming Scopes..........................................................................................................................248
10.7. User−Defined Data Types..........................................................................................................249
10.7.1. Typedefs......................................................................................................................249
10.7.1.1. Mapping typedefs to Java........................................................................................249
10.7.2. Arrays.........................................................................................................................249
10.7.2.1. Mapping arrays to Java............................................................................................249
10.7.3. Sequences....................................................................................................................250
10.7.3.1. Mapping sequences to Java......................................................................................250
10.7.4. Structs........................................................................................................................250
10.7.4.1. Mapping structs to Java...........................................................................................251
10.7.5. Enumerations..............................................................................................................252
10.7.5.1. Mapping enumerations to Java................................................................................253
10.7.6. Unions.........................................................................................................................254
10.7.6.1. Mapping unions to Java...........................................................................................254
10.8. Exceptions..................................................................................................................................254
10.8.1. Standard Exceptions...................................................................................................255
10.8.2. Mapping Exceptions to Java.......................................................................................255
10.9. Module Declarations...................................................................................................................256
10.9.1. Mapping Modules to Java...........................................................................................256
10.10. Interface Declarations...............................................................................................................256
10.10.1. Attributes..................................................................................................................257
10.10.2. Methods....................................................................................................................257
10.10.2.1. Parameters..............................................................................................................258
10.10.2.2. Exceptions..............................................................................................................259
10.10.2.3. Context values........................................................................................................262
10.10.2.4. Call semantics........................................................................................................262
10.10.3. Interface Inheritance.................................................................................................263
10.10.3.1. Method and attribute inheritance...........................................................................264
10.10.3.2. Constant, type, and exception inheritance.............................................................264
10.10.3.3. IDL early binding...................................................................................................265
10.10.4. Mapping Interfaces to Java.......................................................................................265
10.10.4.1. Helper and holder classes......................................................................................266
10.10.4.2. Attributes...............................................................................................................267
10.10.4.3. Methods.................................................................................................................267
11.1. Naming Service..........................................................................................................................268
11.2. Security Service.........................................................................................................................269
11.3. Event Service.............................................................................................................................269
11.4. Persistent Object Service............................................................................................................269
11.5. Life Cycle Service.......................................................................................................................270
11.6. Concurrency Control Service......................................................................................................270
11.7. Externalization Service...............................................................................................................271
11.8. Relationship Service...................................................................................................................271
11.9. Transaction Service.....................................................................................................................272
11.10. Query Service...........................................................................................................................272
11.11. Licensing Service......................................................................................................................274
11.12. Property Service........................................................................................................................274
11.13. Time Service............................................................................................................................275
11.14. Trading Service........................................................................................................................276
11.15. Collection Service.....................................................................................................................276
1. Finding a Quick−Reference Entry...................................................................................................277
2. Reading a Quick−Reference Entry..................................................................................................278
2.1. Class Name, Package Name, Availability, and Flags......................................................278
2.2. Description......................................................................................................................279
2.3. Synopsis..........................................................................................................................280
2.3.1. Member availability and flags......................................................................................281
2.3.2. Functional grouping of members..................................................................................282
2.4. Class Hierarchy................................................................................................................282
2.5. Cross References..............................................................................................................283
2.6. A Note About Class Names.............................................................................................284
Colophon.............................................................................................................................................284
Copyright © 2001 O'Reilly & Associates, Inc. All rights reserved....................................286
Logos and Trademarks............................................................................................................289
Disclaimer..............................................................................................................................299
1. Finding a Quick−Reference Entry...................................................................................................310
2. Reading a Quick−Reference Entry..................................................................................................312
2.1. Class Name, Package Name, Availability, and Flags......................................................315
2.2. Description......................................................................................................................329
2.3. Synopsis..........................................................................................................................356
2.3.1. Member availability and flags......................................................................................365
2.3.2. Functional grouping of members..................................................................................370
2.4. Class Hierarchy................................................................................................................400
2.5. Cross References..............................................................................................................422
2.6. A Note About Class Names.............................................................................................434
Table of Contents....................................................................................................................438
Part 1: Introducing the Java Enterprise APIs.........................................................................446
Part 2: Enterprise Reference..................................................................................................454
Part 3: API Quick Reference.................................................................................................462
Chapter 1. Introduction.................................................................................................................................470
Chapter 2. JDBC............................................................................................................................................474
Chapter 3. Remote Method Invocation.........................................................................................................529
Chapter 4. Java IDL......................................................................................................................................532
Chapter 5. Java Servlets................................................................................................................................534
Chapter 6. JNDI.............................................................................................................................................543
Chapter 7. Enterprise JavaBeans..................................................................................................................545
Chapter 8. SQL Reference............................................................................................................................559
Chapter 9. RMI Tools....................................................................................................................................569
Chapter 10. IDL Reference...........................................................................................................................651
Chapter 11. CORBA Services Reference......................................................................................................651
Chapter 12. Java IDL Tools..........................................................................................................................651
Chapter 13. The java.rmi Package................................................................................................................652
Chapter 14. The java.rmi.activation Package..............................................................................................653
Chapter 15. The java.rmi.dgc Package.........................................................................................................653
Chapter 16. The java.rmi.registry Package..................................................................................................653
Chapter 17. The java.rmi.server Package....................................................................................................656
Chapter 18. The java.sql Package.................................................................................................................657
Chapter 19. The javax.ejb Package...............................................................................................................657
Chapter 20. The javax.ejb.deployment Package..........................................................................................658
Chapter 21. The javax.jms Package..............................................................................................................659
Chapter 22. The javax.naming Package.......................................................................................................659
Chapter 23. The javax.naming.directory Package......................................................................................660
Chapter 24. The javax.naming.spi Package.................................................................................................660
Chapter 25. The javax.servlet Package.........................................................................................................661
Chapter 26. The javax.servlet.http Package.................................................................................................661
Chapter 27. The javax.sql Package...............................................................................................................661
Chapter 28. The javax.transaction Package.................................................................................................662
Chapter 29. The javax.transaction.xa Package............................................................................................663
Chapter 30. The org.omg.CORBA Package.................................................................................................663
Chapter 31. The org.omg.CORBA.DynAnyPackage Package...................................................................663
Chapter 32. The org.omg.CORBA.ORBPackage Package.........................................................................666
Chapter 33. The org.omg.CORBA.portable Package.................................................................................667
Chapter 34. The org.omg.CORBA.TypeCodePackage Package................................................................667
Chapter 35. The org.omg.CosNaming Package...........................................................................................668
Chapter 36. The org.omg.CosNaming. NamingContextPackage Package................................................669
Chapter 37. Class, Method, and Field Index................................................................................................669
How to Use This Quick Reference.................................................................................................................669
How to Use This Quick Reference.................................................................................................................669
Part 1. Introducing the Java Enterprise APIs..............................................................................................671
Part 2. Enterprise Reference.........................................................................................................................672
Part 3. API Quick Reference.........................................................................................................................673
Download
Another Java Books
No comments:
Post a Comment