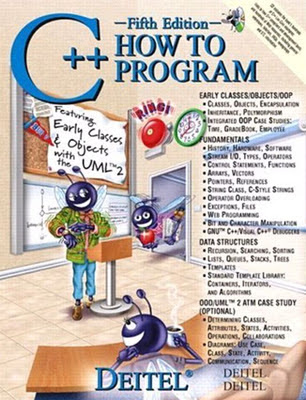
By H. M. Deitel - Deitel & Associates, Inc., P. J. Deitel - Deitel & Associates, Inc.
Publisher : Prentice Hall
Pub Date : January 05, 2005
Print ISBN-10 : 0-13-185757-6
eText ISBN-10 : 0-13-186103-4
Print ISBN-13 : 978-0-13-185757-5
eText ISBN-13 : 978-0-13-186103-9
Pages : 1536
Best-selling C++ text significantly revised to include new early objects coverage and new streamlined case studies.
Copyright iv
Deitel® Books, Cyber Classrooms, Complete Training Courses and Web-Based Training Courses published by Prentice Hall ii
Preface xxiii
Features of C++ How to Program, 5/e xxiii
Teaching Approach xxvii
Tour of the Book xxxi
Object-Oriented Design of an ATM with the UML: A Tour of the Optional Software Engineering Case Study xliv
Software Bundled with C++ How to Program, 5/e xlvi
Teaching Resources for C++ How to Program, 5/e xlix
C++ Multimedia Cyber Classroom, 5/e, Online xlix
C++ in the Lab xlix
CourseCompassSM, WebCT™ and Blackboard™ li
PearsonChoices lii
The Deitel® Buzz Online Free E-mail Newsletter liii
Acknowledgments liii
About the Authors lvi
About Deitel & Associates, Inc. lvii
Before You Begin lix
Resources on the CD That Accompanies C++ How to Program, Fifth Edition lix
Copying and Organizing Files lix
Copying the Book Examples from the CD lx
Changing the Read-Only Property of Files lx
Chapter 1. Introduction to Computers, the Internet and World Wide Web 1
Section 1.1. Introduction 2
Section 1.2. What Is a Computer? 3
Section 1.3. Computer Organization 4
Section 1.4. Early Operating Systems 5
Section 1.5. Personal, Distributed and Client/Server Computing 5
Section 1.6. The Internet and the World Wide Web 6
Section 1.7. Machine Languages, Assembly Languages and High-Level Languages 6
Section 1.8. History of C and C++ 8
Section 1.9. C++ Standard Library 8
Section 1.10. History of Java 9
Section 1.11. FORTRAN, COBOL, Pascal and Ada 10
Section 1.12. Basic, Visual Basic, Visual C++, C# and .NET 11
Section 1.13. Key Software Trend: Object Technology 11
Section 1.14. Typical C++ Development Environment 12
Section 1.15. Notes About C++ and C++ How to Program, 5/e 15
Section 1.16. Test-Driving a C++ Application 16
Section 1.17. Software Engineering Case Study: Introduction to Object Technology and the UML (Required) 22
Section 1.18. Wrap-Up 27
Section 1.19. Web Resources 27
Summary 29
Terminology 31
Self-Review Exercises 33
Answers to Self-Review Exercises 34
Exercises 34
Chapter 2. Introduction to C++ Programming 36
Section 2.1. Introduction 37
Section 2.2. First Program in C++: Printing a Line of Text 37
Section 2.3. Modifying Our First C++ Program 41
Section 2.4. Another C++ Program: Adding Integers 42
Section 2.5. Memory Concepts 46
Section 2.6. Arithmetic 48
Section 2.7. Decision Making: Equality and Relational Operators 51
Section 2.8. (Optional) Software Engineering Case Study: Examining the ATM Requirements Document 56
Section 2.9. Wrap-Up 65
Summary 65
Terminology 67
Self-Review Exercises 68
Answers to Self-Review Exercises 69
Exercises 70
Chapter 3. Introduction to Classes and Objects 74
Section 3.1. Introduction 75
Section 3.2. Classes, Objects, Member Functions and Data Members 75
Section 3.3. Overview of the Chapter Examples 77
Section 3.4. Defining a Class with a Member Function 77
Section 3.5. Defining a Member Function with a Parameter 81
Section 3.6. Data Members, set Functions and get Functions 84
Section 3.7. Initializing Objects with Constructors 91
Section 3.8. Placing a Class in a Separate File for Reusability 95
Section 3.9. Separating Interface from Implementation 99
Section 3.10. Validating Data with set Functions 105
Section 3.11. (Optional) Software Engineering Case Study: Identifying the Classes in the ATM Requirements Document 110
Section 3.12. Wrap-Up 118
Summary 118
Terminology 121
Self-Review Exercises 121
Answers to Self-Review Exercises 122
Exercises 122
Chapter 4. Control Statements: Part 1 124
Section 4.1. Introduction 125
Section 4.2. Algorithms 125
Section 4.3. Pseudocode 126
Section 4.4. Control Structures 127
Section 4.5. if Selection Statement 131
Section 4.6. if...else Double-Selection Statement 132
Section 4.7. while Repetition Statement 137
Section 4.8. Formulating Algorithms: Counter-Controlled Repetition 139
Section 4.9. Formulating Algorithms: Sentinel-Controlled Repetition 145
Section 4.10. Formulating Algorithms: Nested Control Statements 156
Section 4.11. Assignment Operators 161
Section 4.12. Increment and Decrement Operators 161
Section 4.13. (Optional) Software Engineering Case Study: Identifying Class Attributes in the ATM System 165
Section 4.14. Wrap-Up 169
Summary 170
Terminology 172
Self-Review Exercises 173
Answers to Self-Review Exercises 174
Exercises 177
Chapter 5. Control Statements: Part 2 185
Section 5.1. Introduction 186
Section 5.2. Essentials of Counter-Controlled Repetition 186
Section 5.3. for Repetition Statement 188
Section 5.4. Examples Using the for Statement 193
Section 5.5. do...while Repetition Statement 197
Section 5.6. switch Multiple-Selection Statement 199
Section 5.7. break and continue Statements 209
Section 5.8. Logical Operators 211
Section 5.9. Confusing Equality (==) and Assignment (=) Operators 216
Section 5.10. Structured Programming Summary 217
Section 5.11. (Optional) Software Engineering Case Study: Identifying Objects' States and Activities in the ATM System 222
Section 5.12. Wrap-Up 226
Summary 228
Terminology 230
Self-Review Exercises 230
Answers to Self-Review Exercises 231
Exercises 233
Chapter 6. Functions and an Introduction to Recursion 238
Section 6.1. Introduction 239
Section 6.2. Program Components in C++ 240
Section 6.3. Math Library Functions 241
Section 6.4. Function Definitions with Multiple Parameters 243
Section 6.5. Function Prototypes and Argument Coercion 248
Section 6.6. C++ Standard Library Header Files 250
Section 6.7. Case Study: Random Number Generation 252
Section 6.8. Case Study: Game of Chance and Introducing enum 258
Section 6.9. Storage Classes 262
Section 6.10. Scope Rules 265
Section 6.11. Function Call Stack and Activation Records 268
Section 6.12. Functions with Empty Parameter Lists 272
Section 6.13. Inline Functions 273
Section 6.14. References and Reference Parameters 275
Section 6.15. Default Arguments 280
Section 6.16. Unary Scope Resolution Operator 282
Section 6.17. Function Overloading 283
Section 6.18. Function Templates 286
Section 6.19. Recursion 288
Section 6.20. Example Using Recursion: Fibonacci Series 292
Section 6.21. Recursion vs. Iteration 295
Section 6.22. (Optional) Software Engineering Case Study: Identifying Class Operations in the ATM System 298
Section 6.23. Wrap-Up 305
Summary 306
Terminology 309
Self-Review Exercises 311
Answers to Self-Review Exercises 313
Exercises 316
Chapter 7. Arrays and Vectors 326
Section 7.1. Introduction 327
Section 7.2. Arrays 328
Section 7.3. Declaring Arrays 329
Section 7.4. Examples Using Arrays 330
Section 7.5. Passing Arrays to Functions 346
Section 7.6. Case Study: Class GradeBook Using an Array to Store Grades 351
Section 7.7. Searching Arrays with Linear Search 358
Section 7.8. Sorting Arrays with Insertion Sort 359
Section 7.9. Multidimensional Arrays 362
Section 7.10. Case Study: Class GradeBook Using a Two-Dimensional Array 365
Section 7.11. Introduction to C++ Standard Library Class Template vector 372
Section 7.12. (Optional) Software Engineering Case Study: Collaboration Among Objects in the ATM System 377
Section 7.13. Wrap-Up 385
Summary 385
Terminology 387
Self-Review Exercises 388
Answers to Self-Review Exercises 389
Exercises 390
Recursion Exercises 400
vector Exercises 400
Chapter 8. Pointers and Pointer-Based Strings 401
Section 8.1. Introduction 402
Section 8.2. Pointer Variable Declarations and Initialization 403
Section 8.3. Pointer Operators 404
Section 8.4. Passing Arguments to Functions by Reference with Pointers 407
Section 8.5. Using const with Pointers 411
Section 8.6. Selection Sort Using Pass-by-Reference 418
Section 8.7. sizeof Operators 421
Section 8.8. Pointer Expressions and Pointer Arithmetic 424
Section 8.9. Relationship Between Pointers and Arrays 427
Section 8.10. Arrays of Pointers 431
Section 8.11. Case Study: Card Shuffling and Dealing Simulation 432
Section 8.12. Function Pointers 438
Section 8.13. Introduction to Pointer-Based String Processing 443
Section 8.14. Wrap-Up 454
Summary 455
Terminology 456
Self-Review Exercises 457
Answers to Self-Review Exercises 459
Exercises 461
Special Section: Building Your Own Computer 464
More Pointer Exercises 469
String-Manipulation Exercises 474
Special Section: Advanced String-Manipulation Exercises 475
A Challenging String-Manipulation Project 479
Chapter 9. Classes: A Deeper Look, Part 1 480
Section 9.1. Introduction 481
Section 9.2. Time Class Case Study 482
Section 9.3. Class Scope and Accessing Class Members 487
Section 9.4. Separating Interface from Implementation 489
Section 9.5. Access Functions and Utility Functions 491
Section 9.6. Time Class Case Study: Constructors with Default Arguments 493
Section 9.7. Destructors 499
Section 9.8. When Constructors and Destructors Are Called 500
Section 9.9. Time Class Case Study: A Subtle TrapReturning a Reference to a private Data Member 503
Section 9.10. Default Memberwise Assignment 506
Section 9.11. Software Reusability 508
Section 9.12. (Optional) Software Engineering Case Study: Starting to Program the Classes of the ATM System 509
Section 9.13. Wrap-Up 516
Summary 517
Terminology 519
Self-Review Exercises 519
Answers to Self-Review Exercises 520
Exercises 520
Chapter 10. Classes: A Deeper Look, Part 2 523
Section 10.1. Introduction 524
Section 10.2. const (Constant) Objects and const Member Functions 524
Section 10.3. Composition: Objects as Members of Classes 534
Section 10.4. friend Functions and friend Classes 541
Section 10.5. Using the this Pointer 545
Section 10.6. Dynamic Memory Management with Operators new and delete 550
Section 10.7. static Class Members 552
Section 10.8. Data Abstraction and Information Hiding 558
Section 10.9. Container Classes and Iterators 561
Section 10.10. Proxy Classes 562
Section 10.11. Wrap-Up 565
Summary 566
Terminology 567
Self-Review Exercises 568
Answers to Self-Review Exercises 569
Exercises 569
Chapter 11. Operator Overloading; String and Array Objects 571
Section 11.1. Introduction 572
Section 11.2. Fundamentals of Operator Overloading 573
Section 11.3. Restrictions on Operator Overloading 574
Section 11.4. Operator Functions as Class Members vs. Global Functions 576
Section 11.5. Overloading Stream Insertion and Stream Extraction Operators 577
Section 11.6. Overloading Unary Operators 581
Section 11.7. Overloading Binary Operators 581
Section 11.8. Case Study: Array Class 582
Section 11.9. Converting between Types 594
Section 11.10. Case Study: String Class 595
Section 11.11. Overloading ++ and -- 607
Section 11.12. Case Study: A Date Class 609
Section 11.13. Standard Library Class string 613
Section 11.14. explicit Constructors 617
Section 11.15. Wrap-Up 621
Summary 621
Terminology 624
Self-Review Exercises 624
Answers to Self-Review Exercises 625
Exercises 625
Chapter 12. Object-Oriented Programming: Inheritance 633
Section 12.1. Introduction 634
Section 12.2. Base Classes and Derived Classes 635
Section 12.3. protected Members 638
Section 12.4. Relationship between Base Classes and Derived Classes 638
Section 12.5. Constructors and Destructors in Derived Classes 670
Section 12.6. public, protected and private Inheritance 678
Section 12.7. Software Engineering with Inheritance 678
Section 12.8. Wrap-Up 680
Summary 681
Terminology 682
Self-Review Exercises 682
Answers to Self-Review Exercises 683
Exercises 683
Chapter 13. Object-Oriented Programming: Polymorphism 686
Section 13.1. Introduction 687
Section 13.2. Polymorphism Examples 689
Section 13.3. Relationships Among Objects in an Inheritance Hierarchy 690
Section 13.4. Type Fields and switch Statements 707
Section 13.5. Abstract Classes and Pure virtual Functions 708
Section 13.6. Case Study: Payroll System Using Polymorphism 710
Section 13.7. (Optional) Polymorphism, Virtual Functions and Dynamic Binding "Under the Hood" 728
Section 13.8. Case Study: Payroll System Using Polymorphism and Run-Time Type Information with Downcasting, dynamic_cast, typeid and type_info 732
Section 13.9. Virtual Destructors 735
Section 13.10. (Optional) Software Engineering Case Study: Incorporating Inheritance into the ATM System 736
Section 13.11. Wrap-Up 744
Summary 744
Terminology 746
Self-Review Exercises 746
Answers to Self-Review Exercises 747
Exercises 747
Chapter 14. Templates 749
Section 14.1. Introduction 750
Section 14.2. Function Templates 751
Section 14.3. Overloading Function Templates 754
Section 14.4. Class Templates 754
Section 14.5. Nontype Parameters and Default Types for Class Templates 761
Section 14.6. Notes on Templates and Inheritance 762
Section 14.7. Notes on Templates and Friends 762
Section 14.8. Notes on Templates and static Members 763
Section 14.9. Wrap-Up 764
Summary 764
Terminology 765
Self-Review Exercises 766
Answers to Self-Review Exercises 766
Exercises 766
Chapter 15. Stream Input/Output 769
Section 15.1. Introduction 770
Section 15.2. Streams 771
Section 15.3. Stream Output 775
Section 15.4. Stream Input 776
Section 15.5. Unformatted I/O using read, write and gcount 780
Section 15.6. Introduction to Stream Manipulators 781
Section 15.7. Stream Format States and Stream Manipulators 787
Section 15.8. Stream Error States 797
Section 15.9. Tying an Output Stream to an Input Stream 800
Section 15.10. Wrap-Up 800
Summary 800
Terminology 803
Self-Review Exercises 804
Answers to Self-Review Exercises 806
Exercises 808
Chapter 16. Exception Handling 810
Section 16.1. Introduction 811
Section 16.2. Exception-Handling Overview 812
Section 16.3. Example: Handling an Attempt to Divide by Zero 812
Section 16.4. When to Use Exception Handling 819
Section 16.5. Rethrowing an Exception 820
Section 16.6. Exception Specifications 821
Section 16.7. Processing Unexpected Exceptions 822
Section 16.8. Stack Unwinding 823
Section 16.9. Constructors, Destructors and Exception Handling 824
Section 16.10. Exceptions and Inheritance 825
Section 16.11. Processing new Failures 825
Section 16.12. Class auto_ptr and Dynamic Memory Allocation 829
Section 16.13. Standard Library Exception Hierarchy 832
Section 16.14. Other Error-Handling Techniques 834
Section 16.15. Wrap-Up 834
Summary 835
Terminology 837
Self-Review Exercises 838
Answers to Self-Review Exercises 838
Exercises 839
Chapter 17. File Processing 841
Section 17.1. Introduction 842
Section 17.2. The Data Hierarchy 842
Section 17.3. Files and Streams 844
Section 17.4. Creating a Sequential File 845
Section 17.5. Reading Data from a Sequential File 849
Section 17.6. Updating Sequential Files 856
Section 17.7. Random-Access Files 856
Section 17.8. Creating a Random-Access File 857
Section 17.9. Writing Data Randomly to a Random-Access File 862
Section 17.10. Reading from a Random-Access File Sequentially 864
Section 17.11. Case Study: A Transaction-Processing Program 867
Section 17.12. Input/Output of Objects 874
Section 17.13. Wrap-Up 874
Summary 874
Terminology 876
Self-Review Exercises 876
Answers to Self-Review Exercises 877
Exercises 878
Chapter 18. Class string and String Stream Processing 883
Section 18.1. Introduction 884
Section 18.2. string Assignment and Concatenation 885
Section 18.3. Comparing strings 887
Section 18.4. Substrings 890
Section 18.5. Swapping strings 891
Section 18.6. string Characteristics 892
Section 18.7. Finding Strings and Characters in a string 894
Section 18.8. Replacing Characters in a string 896
Section 18.9. Inserting Characters into a string 898
Section 18.10. Conversion to C-Style Pointer-Based char * Strings 899
Section 18.11. Iterators 901
Section 18.12. String Stream Processing 902
Section 18.13. Wrap-Up 905
Summary 906
Terminology 907
Self-Review Exercises 907
Answers to Self-Review Exercises 908
Exercises 908
Chapter 19. Web Programming 911
Section 19.1. Introduction 912
Section 19.2. HTTP Request Types 913
Section 19.3. Multitier Architecture 914
Section 19.4. Accessing Web Servers 915
Section 19.5. Apache HTTP Server 916
Section 19.6. Requesting XHTML Documents 917
Section 19.7. Introduction to CGI 917
Section 19.8. Simple HTTP Transactions 918
Section 19.9. Simple CGI Scripts 920
Section 19.10. Sending Input to a CGI Script 928
Section 19.11. Using XHTML Forms to Send Input 928
Section 19.12. Other Headers 938
Section 19.13. Case Study: An Interactive Web Page 939
Section 19.14. Cookies 943
Section 19.15. Server-Side Files 949
Section 19.16. Case Study: Shopping Cart 954
Section 19.17. Wrap-Up 969
Section 19.18. Internet and Web Resources 969
Summary 970
Terminology 972
Self-Review Exercises 973
Answers to Self-Review Exercises 973
Exercises 974
Chapter 20. Searching and Sorting 975
Section 20.1. Introduction 976
Section 20.2. Searching Algorithms 976
Section 20.3. Sorting Algorithms 982
Section 20.4. Wrap-Up 992
Summary 992
Terminology 994
Self-Review Exercises 994
Answers to Self-Review Exercises 995
Exercises 995
Chapter 21. Data Structures 998
Section 21.1. Introduction 999
Section 21.2. Self-Referential Classes 1000
Section 21.3. Dynamic Memory Allocation and Data Structures 1001
Section 21.4. Linked Lists 1001
Section 21.5. Stacks 1016
Section 21.6. Queues 1021
Section 21.7. Trees 1025
Section 21.8. Wrap-Up 1033
Summary 1034
Terminology 1035
Self-Review Exercises 1036
Answers to Self-Review Exercises 1037
Exercises 1038
Special Section: Building Your Own Compiler 1043
Chapter 22. Bits, Characters, C-Strings and structs 1057
Section 22.1. Introduction 1058
Section 22.2. Structure Definitions 1058
Section 22.3. Initializing Structures 1061
Section 22.4. Using Structures with Functions 1061
Section 22.5. typedef 1061
Section 22.6. Example: High-Performance Card Shuffling and Dealing Simulation 1062
Section 22.7. Bitwise Operators 1065
Section 22.8. Bit Fields 1074
Section 22.9. Character-Handling Library 1078
Section 22.10. Pointer-Based String-Conversion Functions 1084
Section 22.11. Search Functions of the Pointer-Based String-Handling Library 1089
Section 22.12. Memory Functions of the Pointer-Based String-Handling Library 1094
Section 22.13. Wrap-Up 1099
Summary 1099
Terminology 1101
Self-Review Exercises 1102
Answers to Self-Review Exercises 1103
Exercises 1104
Chapter 23. Standard Template Library (STL) 1110
Section 23.1. Introduction to the Standard Template Library (STL) 1112
Section 23.2. Sequence Containers 1124
Section 23.3. Associative Containers 1138
Section 23.4. Container Adapters 1147
Section 23.5. Algorithms 1152
Section 23.6. Class bitset 1183
Section 23.7. Function Objects 1187
Section 23.8. Wrap-Up 1190
Section 23.9. STL Internet and Web Resources 1191
Summary 1192
Terminology 1196
Self-Review Exercises 1197
Answers to Self-Review Exercises 1198
Exercises 1198
Recommended Reading 1199
Chapter 24. Other Topics 1200
Section 24.1. Introduction 1201
Section 24.2. const_cast Operator 1201
Section 24.3. namespaces 1203
Section 24.4. Operator Keywords 1207
Section 24.5. mutable Class Members 1209
Section 24.6. Pointers to Class Members (.* and ->*) 1211
Section 24.7. Multiple Inheritance 1213
Section 24.8. Multiple Inheritance and virtual Base Classes 1218
Section 24.9. Wrap-Up 1222
Section 24.10. Closing Remarks 1223
Summary 1223
Terminology 1225
Self-Review Exercises 1225
Answers to Self-Review Exercises 1226
Exercises 1226
Appendix A. Operator Precedence and Associativity Chart 1228
Section A.1. Operator Precedence 1228
Appendix B. ASCII Character Set 1231
Appendix C. Fundamental Types 1232
Appendix D. Number Systems 1234
Section D.1. Introduction 1235
Section D.2. Abbreviating Binary Numbers as Octal and Hexadecimal Numbers 1238
Section D.3. Converting Octal and Hexadecimal Numbers to Binary Numbers 1239
Section D.4. Converting from Binary, Octal or Hexadecimal to Decimal 1239
Section D.5. Converting from Decimal to Binary, Octal or Hexadecimal 1240
Section D.6. Negative Binary Numbers: Two's Complement Notation 1242
Summary 1243
Terminology 1243
Self-Review Exercises 1244
Answers to Self-Review Exercises 1245
Exercises 1246
Appendix E. C Legacy Code Topics 1247
Section E.1. Introduction 1248
Section E.2. Redirecting Input/Output on UNIX/LINUX/Mac OS X and Windows Systems 1248
Section E.3. Variable-Length Argument Lists 1249
Section E.4. Using Command-Line Arguments 1252
Section E.5. Notes on Compiling Multiple-Source-File Programs 1253
Section E.6. Program Termination with exit and atexit 1255
Section E.7. The volatile Type Qualifier 1257
Section E.8. Suffixes for Integer and Floating-Point Constants 1257
Section E.9. Signal Handling 1257
Section E.10. Dynamic Memory Allocation with calloc and realloc 1260
Section E.11. The Unconditional Branch: goto 1261
Section E.12. Unions 1262
Section E.13. Linkage Specifications 1265
Section E.14. Wrap-Up 1266
Summary 1267
Terminology 1269
Self-Review Exercises 1269
Answers to Self-Review Exercises 1270
Exercises 1270
Appendix F. Preprocessor 1272
Section F.1. Introduction 1273
Section F.2. The #include Preprocessor Directive 1273
Section F.3. The #define Preprocessor Directive: Symbolic Constants 1274
Section F.4. The #define Preprocessor Directive: Macros 1275
Section F.5. Conditional Compilation 1277
Section F.6. The #error and #pragma Preprocessor Directives 1278
Section F.7. The # and ## Operators 1278
Section F.8. Predefined Symbolic Constants 1279
Section F.9. Assertions 1279
Section F.10. Wrap-Up 1280
Summary 1280
Terminology 1281
Self-Review Exercises 1281
Answers to Self-Review Exercises 1282
Exercises 1283
Appendix G. ATM Case Study Code 1285
Section G.1. ATM Case Study Implementation 1285
Section G.2. Class ATM 1286
Section G.3. Class Screen 1293
Section G.4. Class Keypad 1294
Section G.5. Class CashDispenser 1295
Section G.6. Class DepositSlot 1297
Section G.7. Class Account 1298
Section G.8. Class BankDatabase 1300
Section G.9. Class Transaction 1304
Section G.10. Class BalanceInquiry 1306
Section G.11. Class Withdrawal 1308
Section G.12. Class Deposit 1313
Section G.13. Test Program ATMCaseStudy.cpp 1316
Section G.14. Wrap-Up 1317
Appendix H. UML 2: Additional Diagram Types 1318
Section H.1. Introduction 1318
Section H.2. Additional Diagram Types 1318
Appendix I. C++ Internet and Web Resources 1320
Section I.1. Resources 1320
Section I.2. Tutorials 1322
Section I.3. FAQs 1322
Section I.4. Visual C++ 1322
Section I.5. Newsgroups 1323
Section I.6. Compilers and Development Tools 1323
Section I.7. Standard Template Library 1324
Appendix J. Introduction to XHTML 1325
Section J.1. Introduction 1326
Section J.2. Editing XHTML 1326
Section J.3. First XHTML Example 1327
Section J.4. Headers 1330
Section J.5. Linking 1331
Section J.6. Images 1333
Section J.7. Special Characters and More Line Breaks 1338
Section J.8. Unordered Lists 1340
Section J.9. Nested and Ordered Lists 1340
Section J.10. Basic XHTML Tables 1341
Section J.11. Intermediate XHTML Tables and Formatting 1346
Section J.12. Basic XHTML Forms 1349
Section J.13. More Complex XHTML Forms 1352
Section J.14. Internet and World Wide Web Resources 1359
Summary 1359
Terminology 1361
Appendix K. XHTML Special Characters 1363
Appendix L. Using the Visual Studio .NET Debugger 1364
Section L.1. Introduction 1365
Section L.2. Breakpoints and the Continue Command 1365
Section L.3. The Locals and Watch Windows 1371
Section L.4. Controlling Execution Using the Step Into, Step Over, Step Out and Continue Commands 1374
Section L.5. The Autos Window 1377
Section L.6. Wrap-Up 1378
Summary 1379
Terminology 1380
Self-Review Exercises 1380
Answers to Self-Review Exercises 1380
Appendix M. Using the GNU C++ Debugger 1381
Section M.1. Introduction 1382
Section M.2. Breakpoints and the run, stop, continue and print Commands 1382
Section M.3. The print and set Commands 1389
Section M.4. Controlling Execution Using the step, finish and next Commands 1391
Section M.5. The watch Command 1393
Section M.6. Wrap-Up 1396
Summary 1397
Terminology 1398
Self-Review Exercises 1398
Answers to Self-Review Exercises 1398
Bibliography 1399
End User License Agreements EULA-1
Prentice Hall License Agreement and Limited Warranty EULA-1
License Agreement and Limited Warranty EULA-3
Using the CD-ROM EULA-3
Contents of the CD-ROM EULA-3
Software and Hardware System Requirements EULA-3
Index
Another C++ Books
Another Programming Language Books
Download
No comments:
Post a Comment