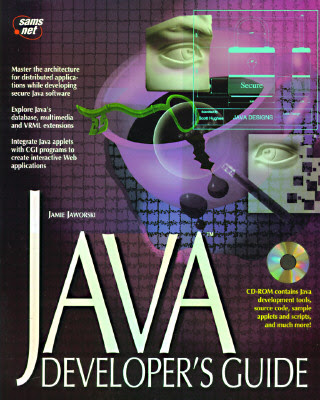
by Jamie Jaworski
C O N T E N T S
Introduction
Chapter 1 The Java Phenomenon
What Is Java?
The Origins of Java
Why Program in Java?
Java and HotJava
Summary
Chapter 2 Java Overview
Getting the JDK
A Quick Tour of the JDK
The Java Language
Java Is Familiar and Simple
Java Is Object-Oriented
Java Is Safer and More Reliable
Java Is Secure
Java Is Multithreaded
Java Is Interpreted and Portable
Java Is the Programming Language of the Web
The Java API
Summary
Chapter 3 Using the Java Developer's Kit
Overview
The Compiler
Using Classes from Other Packages
Setting CLASSPATH
Changing the Root Directory
Generating Debugging Tables
Code Optimization
Suppressing Warnings
Using Verbose Mode
The Interpreter
Changing CLASSPATH
Checking for Source Code Changes
Verifying Your Code
Controlling the Garbage Collector
Changing Properties
Setting Memory and Stack Limits
Debugging Options
The Debugger
The Disassembler
The Applet Viewer
Automating Software Documentation
Header File Generation
Running the Demo Programs
Summary
Chapter 4 First Programs: Hello World! to BlackJack
Hello World!
Comments
Java Program Structure
The package Statement
The imprt Statement
Classes and Methods
The System Class
I Can Read!
Overview of ICanReadApp
Declaring Variables and Creating Objects
Identifiers and Keywords
Using System.in
Type This!
Overview of TypeThisApp
The Primitive Java Data Types
Literal Values
BlackJack
Overview of BlackJackApp
Arrays
Statements
Summary
Chapter 5 Classes and Objects
Object-Oriented Programming Concepts
It's an Object-Oriented World
Composition and Reuse
Classes
Classification and Inheritance
Multiple Inheritance
Messages, Methods, and Object Interaction
Encapsulation
Polymorphism
Dynamic Binding
Java Classes
Class Syntax
The Point Class
Class Modifiers
Extending Superclasses
Adding Body to Classes
The CGrid Class
The CGObject Class
The PrintCGrid Class
The BorderedPrintCGrid Class
The CGPoint Class
The CGBox Class
The CGText Class
The KeyboardInput Class
The CDrawApp Program
Running CDrawApp
CDrawApp's Implementation of Object-Oriented Concepts
Summary
Chapter 6 Interfaces
The Purpose of Java Interfaces
The Benefits of Interfaces
Declaring Interfaces
Implementing Interfaces
The CDrawApp Interface Example
The CGTextEdit Interface
Updating the CGText Class
The CGTextPoint Class
The CGTextBox Class
Updating the CDraw Class
Running the Example
Example Summary
Using Interfaces as Abstract Types
Interface Constants
Extending Interfaces
Combining Interfaces
Summary
Chapter 7 Exceptions
Eliminating Software Errors
Error Processing and Exceptions
Throwing Exceptions
Declaring Exceptions
Declare or Catch?
Using the try Statement
Catching Exceptions
Nested Exception Handling
Rethrowing Exceptions
Analysis of NestedExceptionTest
Summary
Chapter 8 Multithreading
Understanding Multithreading
How Java Supports Multithreading
Creating Subclasses of Thread
Implementing Runnable
Thread States
Thread Priority and Scheduling
Synchronization
Daemon Threads
Thread Groups
Summary
Chapter 9 Using the Debugger
Overview of the Debugger
An Extended Example
Debugging Multithreaded Programs
Summary
Chapter 10 Automating Software Documentation
How javadoc Works
Using javadoc
Placing Doc Comments
Using javadoc Tags
Embedding Standard HTML
Summary
Chapter 11 Language Summary
The package Statement
The imprt Statement
Comments
Identifiers
Reserved Words
Primitive Data Types and Literal Values
Class Declarations
Variable Declarations
Constructor Declarations
Access Method Declarations
Static Initializers
Interfaces
Blocks and Block Bodies
Local Variable Declarations
Statements
Empty Statement
Block Statement
Method Invocation
Allocation Statements
Assignment Statements
The if Statement
Statement Labels
The switch Statement
The break Statement
The for Statement
The while Statement
The do Statement
The continue Statement
The synchronized Statement
The try Statement
The return Statement
Operators
Summary
Chapter 12 Portable Software and the java.lang Package
The Object and Class Classes
Object
Class
A Touch of Class
The ClassLoader, SecurityManager, and Runtime Classes
ClassLoader
SecurityManager
Runtime
The System Class
Property-Related Methods
Security Manager-Related Methods
Runtime-Related Methods
Odds and Ends
Time and Properties
Wrapped Classes
The Boolean Class
The Character Class
The Integer and Long Classes
The Double and Float Classes
The Number Class
All Wrapped Up
The Math Class
The String and StringBuffer Classes
String Literals
The + Operator and StringBuffer
String Constructors
String Access Methods
The StringBuffer Class
Threads and Processes
Runnable
Thread
ThreadGroup
Process
Hello Again
The Compiler Class
Exceptions and Errors
The Throwable Class
The Error Class
The Exception Class
Summary
Chapter 13 Stream-Based Input/Output and the java.io Package
Streams
The java.io Class Hierarchy
The InputStream Class
The read() Method
The available() Method
The close() Method
Markable Streams
The skip() Method
The OutputStream Class
The write() Method
The flush() Method
The close() Method
Byte Array I/O
The ByteArrayInputStream Class
The ByteArrayOutputStream Class
The ByteArrayIOApp Program
The StringBufferInputStream Class
File I/O
The File Class
The FileDescriptor Class
The FileInputStream Class
The FileOutputStream Class
The FileIOApp Program
The SequenceInputStream Class
The SequenceIOApp Program
Filtered I/O
The FilterInputStream Class
The FilterOutputStream Class
Buffered I/O
PushbackInputStream
The LineNumberInputStream Class
Data I/O
The PrintStream Class
Piped I/O
The RandomAccessFile Class
The RandomIOApp Program
The StreamTokenizer Class
The StreamTokenApp Program
Summary
Chapter 14 Useful Tools in the java.util Package
The Date Class
DateApp
The Random Class
RandomApp
The Enumeration Interface
The Vector Class
VectorApp
The Stack Class
StackApp
The BitSet Class
BitSetApp
The Dictionary, Hashtable, and Properties Classes
Dictionary
Hashtable
The Properties Class
The StringTokenizer Class
TokenApp
Observer and Observable
Observable
Summary
Chapter 15 Window Programming with the java.awt Package
Window Programming Classes
Components and Containers
The Container Class
The Window Class
The Panel Class
The Label Class
The Button Class
The Checkbox Class
The Choice Class
The List Class
The TextComponent Class
The Canvas Class
The Scrollbar Class
Constructing Menus
The MenuBar Class
The MenuItem Class
The Menu Class
The CheckboxMenuItem Class
The MenuContainer Class
Organizing Windows
The LayoutManager Class
The BorderLayout Class
The CardLayout Class
The FlowLayout Class
The GridLayout Class
The GridBagLayout Class
Handling Events
Working with Images
The Color Class
The java.awt.image Package
The MediaTracker Class
Geometrical Objects
The Point Class
The Rectangle Class
The Polygon Class
The Dimension Class
Using Fonts
The FontMetrics Class
Using the Toolkit
Summary
Chapter 16 Web Programming With the java.applet Package
Applets and the World Wide Web
The Applet Class
Applets and HTML
The Life Cycle of an Applet
Responding to Events
Using Window Components
Adding Audio and Animation
Summary
Chapter 17 Network Programming with the java.net Package
The Internet Protocol Suite
What Is the Internet and How Does It Work?
Connection-Oriented Versus Connectionless Communication
Client/Server Computing and the Internet
Sockets and Client/Server Communication
Overview of java.net
The InetAddress Class
The Socket Class
The ServerSocket Class
The DatagramSocket Class
The DatagramPacket Class
TimeServerApp
GetTimeApp
The SocketImpl Class and the SocketImplFactory Interface
Web-Related Classes
URL
URLConnection
URLEncoder
The ContentHandler and ContentHandlerFactory Classes
The URLStreamHandler Class and the URLStreamHandlerFactory Interface
Summary
Chapter 18 Opening Windows
Hello Windows!
Going Round in Ovals: A Graphics Program
A Text Editor
Summary
Chapter 19 Organizing Window Programs
Designing Window Programs
Opening and Closing Windows
Using Layouts
Connecting Code to Events
The Window Sampler Program
MyTextField
MyButton
MyCanvas
MyCheckBoxGroup
MyChoice
MyList
MyScrollbar
Summary
Chapter 20 Menus, Buttons, and Dialong Boxes
Adding Menus to Windows
The MyMenu Class
The MyMenuBar Class
The MenuApp Program
Working with Buttons
The ButtonBar Class
The ButtonApp Program
Using Dialog Boxes
The MessageDialog Class
The MessageApp Program
The FileDialog Class
Summary
Chapter 21 Checkboxes, Choices, and Lists
Using Checkboxes
The CheckboxPanel Class
Working with Radio Buttons
The CheckboxGroupPanel Class
The CheckboxApp Program
Making Choices
The MyChoice Class
Selecting from Lists
The MyList Class
The ChoiceListApp Program
Summary
Chapter 22 Text and Fonts
The Text Classes
Font Basics
Using the Toolkit Class
The FontApp Program
WYSIWYG Editors
The EditApp Program
The FontDialog Class
The ColorDialog Class
Summary
Chapter 23 The Canvas
The Canvas and Graphics Classes
Displaying Bitmapped Images
The DisplayImageApp Program
Drawing and Painting
The DrawApp Program
Combining Graphics and Text
The Image-Processing Classes of java.awt.image
The ImageApp Program
Summary
Chapter 24 Scrollbars
How Scrollbars Work
Using Scrollbars
Scrolling Text
The TextScrollApp Program
Scrolling Graphics
The ImageScrollApp Program
Summary
Chapter 25 Using Animation
Animation Basics
A Simple Animation
A Graphics Animation
Improving Animation Display Qualities
An Updated Graphics Animation
Summary
Chapter 26 Client Programs
Types of Clients
Client Responsibilities
A Simple Telnet Client
The Network Virtual Terminal
The Interpret as Command Code
Negotiated Options
Symmetry Between Terminals and Processes
The TelnetApp Program
The NVTPrinter Class
The NVTInputStream Class
The NVTOutputStream Class
A Mail Client
The MailClientApp Program
The Web Fetcher Program
Summary
Chapter 27 Server Programs
Types of Servers
Server Responsibilities
An SMTP Server
The SMTPServerApp Program
A Web Server
The WebServerApp Program
Summary
Chapter 28 Content Handlers
Using Content Handlers
Multipurpose Internet Mail Extensions (MIME)
Developing a Content Handler
A Simple Content Handler
The GridContentHandler Class
The GetGridApp Program
Summary
Chapter 29 Protocol Handlers
Using Protocol Handlers
Developing a Protocol Handler
A Simple Protocol Handler
Summary
Chapter 30 Sample Applets
Hello Web!
An Audio Player
BlackJack Revisited
Summary
Chapter 31 Developing Applets
How Applets Work
The Relationship Between HTML and Applets
Applets and Interactive Web Pages
Applets Versus Scripts
The Execution of an Applet
Using Window Components
Adding Content and Protocol Handlers to Applets
Using Applets as Inline Viewers
Using Applets as Network Clients
Optimizing Applets
Learning from Online Examples
Summary
Chapter 32 Integrating Applets into Distributed Applications
Architecture for Distributed Applications
OMG and CORBA
The Spring Project and Java IDL
NEO and JOE
JIDL
Black Widow
HORB
Interfacing with CGI Programs
How CGI Programs Work
Connecting Applets with CGI Programs
Fortune Teller
Summary
Chapter 33 Working with JavaScript
JavaScript and Java
How JavaScript Works
The Relationship Between HTML and Scripts
Embedding JavaScript
Combining Scripts with Applets
Summary
Chapter 34 Sample Scripts
A Customizable Web Page
Story Teller
Web Guide
Summary
Chapter 35 JavaScript Reference
JavaScript Objects
Properties and Methods
The navigator Object
The window Object
The location Object
The history Object
The document Object
The form Object
The button Object
The checkbox Object
The text, textarea, hidden, and password Objects
The radio Object
The select Object
The string Object
The Date Object
The Math Object
Handling Events
Arrays
Operators and Expressions
Statements
Summary
Chapter 36 The Java Source Code
Obtaining the Java Source Code
Platform Differences
Platform Benchmarks
Windows 95/NT Overview
Summary
Chapter 37 The Java Virtual Machine
Overview
Structure of .class Files
Magic
Version
Constant_pool
Access_flags
This_class
Interfaces
Fields
Methods
Attributes
Virtual Machine Architecture
JVM Registers
JVM Stack
Garbage-Collected Heap
Method Area
Instruction Set
Pushing Constants onto the Stack
Pushing Local Variables onto the Stack
Storing Stack Values into Local Variables
Managing Arrays
Stack Instructions
Arithmetic Instructions
Logical Instructions
Conversion Operations
Control Transfer Instructions
Function Return Instructions
Table Jumping Instructions
Manipulating Object Fields
Method Invocation
Exception Handling
Object Utility Operations
Monitors
The breakpoint Instruction
Summary
Chapter 38 Creating Native Methods
Using Native Methods
How Native Methods Are Called
How Native Methods Are Created
Summary
Chapter 39 Java Security
Threats to Java Security
Java Security Features
Language Security Features
Compiler Security Features
Runtime Security Mechanisms
Staying Current with Java Security Issues
Summary
Chapter 40 Java Platforms and Extensions
Java-Enabled Browsers
HotJava
Netscape Navigator
Oracle PowerBrowser
Borland's Latte
Visual Design
The Integrated Development Environment
Performance Improvements
Symantec's Café
Database Extensions
VRML Extensions with Iced Java/Liquid Reality
Three-Dimensional Modeling
Iced Java and Liquid Reality
Summary
Appendix A The Jawa API Quick Reference
java.applet
java.awt
java.awt.image
java.awt.peer
java.io
java.lang
java.net
java.util
Appendix B Differences Between java and C++
Program Structure
The main() Method
Packages
Imprting Classes
Functions and Variables Declared Outside of Classes
Program Development
Compilation and Execution
The Preprocessor and Compiler Directives
The Java API Versus C++ Libraries
Using CLASSPATH
Language Syntax
Comments
Constants
Primitive Data Types
Keywords
Java Classes Versus C++ Classes
Public, Private, and Protected Keywords
Variables
Types
Pointers
Objects
Arrays
Strings
null Versus NULL
Statements
Methods Versus Functions
Operators
Appendix C Moving C/C++ Legacy Code to Java
Why Move to Java?
Platform Independence
Object Orientation
Security
Reliability
Simplicity
Language Features
Standardization
The Java API
Transition to Distributed Computing
Rapid Code Generation
Ease of Documentation and Maintenance
Reasons Against Moving to Java
Compatibility
Performance
Retraining
Impact on Existing Operations
Cost, Schedule, and Level of Effort
Transition Approaches and Issues
Interfacing with Existing Legacy Cod
Incremental Reimplementation of Legacy Code
Off-Boarding Access to Legacy Objects
Full-Scale Redevelopment
Translation Approaches and Issues
Automated Translation
Manual Translation
Source-Level Redesign
Credits
Another Java Books
Another Programming Language
Download
No comments:
Post a Comment