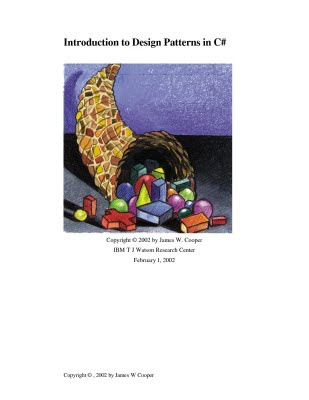
1. What are Design Patterns? ............................................................ 21
Defining Design Patterns ...................................................................... 23
The Learning Process............................................................................ 25
Studying Design Patterns ...................................................................... 26
Notes on Object-Oriented Approaches ................................................. 26
C# Design Patterns................................................................................ 27
How This Book Is Organized ............................................................... 28
2. Syntax of the C# Language ............................................................ 29
Data Types ............................................................................................ 30
Converting Between Numbers and Strings ........................................... 32
Declaring Multiple Variables................................................................ 32
Numeric Constants ................................................................................ 32
Character Constants .............................................................................. 33
Variables ............................................................................................... 33
Declaring Variables as You Use Them............................................. 34
Multiple Equals Signs for Initialization................................................ 34
A Simple C# Program........................................................................... 34
Compiling & Running This Program................................................ 36
Arithmetic Operators............................................................................. 36
Increment and Decrement Operators .................................................... 37
Combining Arithmetic and Assignment Statements ............................. 37
Making Decisions in C#........................................................................ 38
Comparison Operators .......................................................................... 39
Combining Conditions .......................................................................... 39
The Most Common Mistake ................................................................. 40
The switch Statement ............................................................................ 41
C# Comments........................................................................................ 41
The Ornery Ternary Operator ............................................................... 42
Looping Statements in C#..................................................................... 42
The while Loop ..................................................................................... 42
The do-while Statement ........................................................................ 43
The for Loop ......................................................................................... 43
Declaring Variables as Needed in For Loops ....................................... 44
Commas in for Loop Statements........................................................... 44
How C# Differs From C ....................................................................... 45
Summary............................................................................................... 46
3. Writing Windows C# Programs ................................................... 47
Objects in C#......................................................................................... 47
Managed Languages and Garbage Collection ...................................... 48
Classes and Namespaces in C# ............................................................. 48
Building a C# Application .................................................................... 49
The Simplest Window Program in C# .................................................. 50
Windows Controls ................................................................................ 54
Labels ................................................................................................ 55
TextBox............................................................................................. 55
CheckBox.......................................................................................... 56
Buttons .............................................................................................. 56
Radio buttons .................................................................................... 56
Listboxes and Combo Boxes ............................................................ 57
The Items Collection......................................................................... 57
Menus................................................................................................ 58
ToolTips............................................................................................ 58
Other Windows Controls .................................................................. 59
The Windows Controls Program .......................................................... 59
Summary............................................................................................... 61
Programs on the CD-ROM ................................................................... 47
4. Using Classes and Objects in C# .................................................... 62
What Do We Use Classes For? ............................................................. 62
A Simple Temperature Conversion Program........................................ 62
Building a Temperature Class............................................................... 64
Converting to Kelvin......................................................................... 67
Putting the Decisions into the Temperature Class ................................ 67
Using Classes for Format and Value Conversion................................. 68
Handling Unreasonable Values......................................................... 71
A String Tokenizer Class ...................................................................... 71
Classes as Objects ................................................................................. 73
Class Containment ............................................................................ 75
Initialization.......................................................................................... 76
Classes and Properties........................................................................... 77
Programming Style in C#...................................................................... 79
Summary............................................................................................... 80
Programs on the CD-ROM ................................................................... 62
5. Inheritance ....................................................................................... 81
Constructors .......................................................................................... 81
Drawing and Graphics in C#................................................................. 82
Using Inheritance .................................................................................. 84
Namespaces........................................................................................... 85
Creating a Square From a Rectangle ................................................. 86
Public, Private and Protected ................................................................ 88
Overloading........................................................................................... 89
Virtual and Override Keywords ............................................................ 89
Overriding Methods in Derived Classes ............................................... 90
Replacing Methods Using New ............................................................ 91
Overriding Windows Controls .............................................................. 92
Interfaces ............................................................................................... 94
Abstract Classes .................................................................................... 95
Comparing Interfaces and Abstract Classes.......................................... 97
Summary............................................................................................... 99
Programs on the CD-ROM ................................................................... 99
6. UML Diagrams .............................................................................. 100
Inheritance........................................................................................... 102
Interfaces ............................................................................................. 103
Composition........................................................................................ 103
Annotation........................................................................................... 105
WithClass UML Diagrams ................................................................. 106
C# Project Files ................................................................................... 106
7. Arrays, Files and Exceptions in C# ............................................. 107
Arrays.................................................................................................. 107
Collection Objects............................................................................... 108
ArrayLists........................................................................................ 108
Hashtables ....................................................................................... 109
SortedLists ...................................................................................... 110
Exceptions ........................................................................................... 110
Multiple Exceptions ............................................................................ 112
Throwing Exceptions .......................................................................... 113
File Handling....................................................................................... 113
The File Object................................................................................ 113
Reading Text File........................................................................... 114
Writing a Text File .......................................................................... 114
Exceptions in File Handling................................................................ 114
Testing for End of File ........................................................................ 115
A csFile Class...................................................................................... 116
8. The Simple Factory Pattern......................................................... 121
How a Simple Factory Works ............................................................. 121
Sample Code ....................................................................................... 122
The Two Derived Classes ................................................................... 122
Building the Simple Factory............................................................... 123
Using the Factory............................................................................ 124
Factory Patterns in Math Computation............................................... 125
Programs on the CD-ROM ................................................................. 128
Thought Questions .............................................................................. 128
9. The Factory Method ..................................................................... 129
The Swimmer Class ............................................................................ 132
The Events Classes.............................................................................. 132
Straight Seeding .................................................................................. 133
Circle Seeding ................................................................................. 134
Our Seeding Program.......................................................................... 134
Other Factories .................................................................................... 135
When to Use a Factory Method .......................................................... 136
Thought Question................................................................................ 136
Programs on the CD-ROM ................................................................. 136
10. The Abstract Factory Pattern.................................................. 137
A GardenMaker Factory ..................................................................... 137
The PictureBox ............................................................................... 141
Handling the RadioButton and Button Events ................................ 142
Adding More Classes.......................................................................... 143
Consequences of Abstract Factory...................................................... 144
Thought Question................................................................................ 144
Programs on the CD-ROM ................................................................. 144
11. The Singleton Pattern ............................................................... 145
Creating Singleton Using a Static Method.......................................... 145
Exceptions and Instances .................................................................... 146
Throwing the Exception...................................................................... 147
Creating an Instance of the Class ........................................................ 147
Providing a Global Point of Access to a Singleton............................. 148
Other Consequences of the Singleton Pattern..................................... 149
Programs on Your CD-ROM.............................................................. 149
12. The Builder Pattern .................................................................. 150
An Investment Tracker........................................................................ 151
The Stock Factory........................................................................... 154
The CheckChoice Class .................................................................. 155
The ListboxChoice Class ................................................................ 156
Using the Items Collection in the ListBox Control ............................ 157
Plotting the Data.............................................................................. 158
The Final Choice ............................................................................. 159
Consequences of the Builder Pattern.................................................. 160
Thought Questions .............................................................................. 161
Programs on the CD-ROM ................................................................. 161
13. The Prototype Pattern .............................................................. 162
Cloning in C# ...................................................................................... 163
Using the Prototype............................................................................. 163
Cloning the Class ................................................................................ 167
Using the Prototype Pattern ................................................................ 170
Dissimilar Classes with the Same Interface .................................... 172
Prototype Managers ............................................................................ 176
Consequences of the Prototype Pattern............................................... 176
Thought Question................................................................................ 177
Programs on the CD-ROM ................................................................. 177
Summary of Creational Patterns ......................................................... 178
14. The Adapter Pattern................................................................. 180
Moving Data Between Lists................................................................ 180
Making an Adapter.............................................................................. 182
Using the DataGrid ............................................................................. 183
Detecting Row Selection................................................................. 186
Using a TreeView ............................................................................... 186
The Class Adapter ............................................................................... 188
Two-Way Adapters............................................................................. 190
Object Versus Class Adapters in C# ................................................... 190
Pluggable Adapters ............................................................................. 191
Thought Question................................................................................ 191
Programs on the CD-ROM ................................................................. 191
15. The Bridge Pattern.................................................................... 192
The VisList Classes............................................................................. 195
The Class Diagram.............................................................................. 196
Extending the Bridge .......................................................................... 197
Windows Forms as Bridges ................................................................ 201
Consequences of the Bridge Pattern ................................................... 202
Thought Question................................................................................ 203
Programs on the CD-ROM ................................................................. 203
16. The Composite Pattern............................................................. 204
An Implementation of a Composite .................................................... 205
Computing Salaries............................................................................. 206
The Employee Classes ........................................................................ 206
The Boss Class.................................................................................... 209
Building the Employee Tree ............................................................... 210
Self-Promotion.................................................................................... 213
Doubly Linked Lists ........................................................................... 213
Consequences of the Composite Pattern............................................. 215
A Simple Composite ........................................................................... 215
Composites in .NET............................................................................ 216
Other Implementation Issues .............................................................. 216
Thought Questions .............................................................................. 216
Programs on the CD-ROM ................................................................. 217
17. The Decorator Pattern.............................................................. 218
Decorating a CoolButton .................................................................... 218
Handling events in a Decorator........................................................... 220
Layout Considerations .................................................................... 221
Control Size and Position................................................................ 221
Multiple Decorators ............................................................................ 222
Nonvisual Decorators.......................................................................... 225
Decorators, Adapters, and Composites ............................................... 226
Consequences of the Decorator Pattern.............................................. 226
Thought Questions .............................................................................. 226
Programs on the CD-ROM ................................................................. 227
18. The Façade Pattern................................................................... 228
What Is a Database? ............................................................................ 228
Getting Data Out of Databases............................................................ 230
Kinds of Databases.............................................................................. 231
ODBC.................................................................................................. 232
Database Structure .............................................................................. 232
Using ADO.NET................................................................................. 233
Connecting to a Database................................................................ 233
Reading Data from a Database Table ............................................. 234
dtable = dset.Tables [0]; ............................................................ 235
Executing a Query........................................................................... 235
Deleting the Contents of a Table ..................................................... 235
Adding Rows to Database Tables Using ADO.NET .......................... 236
Building the Façade Classes ............................................................... 237
Building the Price Query................................................................. 239
Making the ADO.NET Façade............................................................ 239
The DBTable class.......................................................................... 242
Creating Classes for Each Table ......................................................... 244
Building the Price Table ..................................................................... 246
Loading the Database Tables .............................................................. 249
The Final Application ......................................................................... 251
What Constitutes the Façade? ............................................................. 252
Consequences of the Façade ............................................................... 253
Thought Question................................................................................ 253
Programs on the CD-ROM ................................................................. 253
19. The Flyweight Pattern .............................................................. 254
Discussion........................................................................................... 255
Example Code ..................................................................................... 256
The Class Diagram.......................................................................... 261
Selecting a Folder............................................................................ 261
Handling the Mouse and Paint Events ................................................ 263
Flyweight Uses in C#.......................................................................... 264
Sharable Objects ................................................................................. 265
Copy-on-Write Objects....................................................................... 265
Thought Question................................................................................ 266
Programs on the CD-ROM ................................................................. 266
20. The Proxy Pattern..................................................................... 267
Sample Code ....................................................................................... 268
Proxies in C# ....................................................................................... 270
Copy-on-Write .................................................................................... 271
Comparison with Related Patterns ...................................................... 271
Thought Question................................................................................ 271
Programs on the CD-ROM ................................................................. 271
21. Chain of Responsibility............................................................. 274
Applicability........................................................................................ 275
Sample Code ....................................................................................... 276
The List Boxes .................................................................................... 280
Programming a Help System .............................................................. 282
Receiving the Help Command ........................................................ 286
A Chain or a Tree? .............................................................................. 287
Kinds of Requests ............................................................................... 289
Examples in C# ................................................................................... 289
Consequences of the Chain of Responsibility .................................... 290
Thought Question................................................................................ 290
Programs on the CD-ROM ................................................................. 291
22. The Command Pattern ............................................................. 292
Motivation........................................................................................... 292
Command Objects............................................................................... 293
Building Command Objects................................................................ 294
Consequences of the Command Pattern ............................................. 297
The CommandHolder Interface .......................................................... 297
Providing Undo ................................................................................... 301
Thought Questions .............................................................................. 309
Programs on the CD-ROM ................................................................. 310
23. The Interpreter Pattern............................................................ 311
Motivation........................................................................................... 311
Applicability........................................................................................ 311
A Simple Report Example .................................................................. 312
Interpreting the Language ................................................................... 314
Objects Used in Parsing ...................................................................... 315
Reducing the Parsed Stack .................................................................. 319
Implementing the Interpreter Pattern.................................................. 321
The Syntax Tree .............................................................................. 322
Consequences of the Interpreter Pattern ............................................. 326
Thought Question................................................................................ 327
Programs on the CD-ROM ................................................................. 327
24. The Iterator Pattern.................................................................. 328
Motivation........................................................................................... 328
Sample Iterator Code .......................................................................... 329
Fetching an Iterator ......................................................................... 330
Filtered Iterators .................................................................................. 331
The Filtered Iterator ........................................................................ 331
Keeping Track of the Clubs ................................................................ 334
Consequences of the Iterator Pattern .................................................. 335
Programs on the CD-ROM ................................................................. 336
25. The Mediator Pattern ............................................................... 337
An Example System............................................................................ 337
Interactions Between Controls ............................................................ 339
Sample Code ....................................................................................... 341
Initialization of the System ............................................................. 345
Mediators and Command Objects....................................................... 345
Consequences of the Mediator Pattern................................................ 347
Single Interface Mediators.................................................................. 348
Implementation Issues......................................................................... 349
Programs on the CD-ROM ................................................................. 349
26. The Memento Pattern............................................................... 350
Motivation........................................................................................... 350
Implementation ................................................................................... 351
Sample Code ....................................................................................... 351
A Cautionary Note .......................................................................... 358
Command Objects in the User Interface ............................................. 358
Handling Mouse and Paint Events ...................................................... 360
Consequences of the Memento ........................................................... 361
Thought Question................................................................................ 361
Programs on the CD-ROM ................................................................. 362
27. The Observer Pattern ............................................................... 363
Watching Colors Change .................................................................... 364
The Message to the Media .................................................................. 367
Consequences of the Observer Pattern................................................ 368
Programs on the CD-ROM ................................................................. 369
28. The State Pattern ...................................................................... 370
Sample Code ....................................................................................... 370
Switching Between States ................................................................... 376
How the Mediator Interacts with the State Manager .......................... 377
The ComdToolBarButton ............................................................... 378
Handling the Fill State ........................................................................ 381
Handling the Undo List....................................................................... 382
The VisRectangle and VisCircle Classes............................................ 385
Mediators and the God Class .............................................................. 387
Consequences of the State Pattern...................................................... 388
State Transitions .................................................................................. 388
Thought Questions .............................................................................. 389
Programs on the CD-ROM ................................................................. 389
29. The Strategy Pattern................................................................. 390
Motivation........................................................................................... 390
Sample Code ....................................................................................... 391
The Context......................................................................................... 392
The Program Commands .................................................................... 393
The Line and Bar Graph Strategies..................................................... 394
Drawing Plots in C#............................................................................ 394
Making Bar Plots ............................................................................ 395
Making Line Plots ........................................................................... 396
Consequences of the Strategy Pattern................................................. 398
Programs on the CD-ROM ................................................................. 398
30. The Template Method Pattern ................................................ 399
Motivation........................................................................................... 399
Kinds of Methods in a Template Class ............................................... 401
Sample Code ....................................................................................... 402
Drawing a Standard Triangle .......................................................... 404
Drawing an Isosceles Triangle ........................................................ 404
The Triangle Drawing Program.......................................................... 405
Templates and Callbacks .................................................................... 406
Summary and Consequences .............................................................. 407
Programs on the CD-ROM ................................................................. 408
31. The Visitor Pattern ................................................................... 409
Motivation........................................................................................... 409
When to Use the Visitor Pattern ......................................................... 411
Sample Code ....................................................................................... 411
Visiting the Classes ............................................................................. 413
Visiting Several Classes...................................................................... 414
Bosses Are Employees, Too ............................................................... 416
Catch-All Operations with Visitors .................................................... 417
Double Dispatching............................................................................. 419
Why Are We Doing This? .................................................................. 419
Traversing a Series of Classes ............................................................ 419
Consequences of the Visitor Pattern................................................... 420
Thought Question................................................................................ 420
Programs on the CD-ROM ................................................................. 421
32. Bibliography .............................................................................. 422
Another C# Books
Another Programming Language Books
Another Software Engineering Books
Download
No comments:
Post a Comment