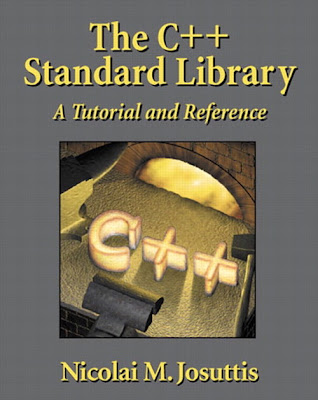
C++ Standard Library provides a set of common classes and interfaces that greatly extend the core C++ language. The library, however, is not self-explanatory. To make full use of its components-and to benefit from their power-you need a resource that does far more than list the classes and their functions.
The C++ Standard Library not only provides comprehensive documentation of each library component, it also offers clearly written explanations of complex concepts, describes the practical programming details needed for effective use, and gives example after example of working code.
This thoroughly up-to-date book reflects the newest elements of the C++ standard library incorporated into the full ANSI/ISO C++ language standard. In particular, the text focuses on the Standard Template Library (STL), examining containers, iterators, function objects, and STL algorithms. You will also find detailed coverage of special containers, strings, numerical classes, internationalization, and the IOStream library. Each component is presented in depth, with an introduction to its purpose and design, examples, a detailed description, traps and pitfalls, and the exact signature and definition of its classes and their functions. An insightful introduction to fundamental concepts and an overview of the library will help bring newcomers quickly up to speed.
Comprehensive, detailed, readable, and practical, The C++ Standard Library is the C++ resource you will turn to again and again.
Preface
Acknowledgments
Chapter 1. About this Book
1.1 Why this Book
1.2 What You Should Know Before Reading this Book
1.3 Style and Structure of the Book
1.4 How to Read this Book
1.5 State of the Art
1.6 Example Code and Additional Information
1.7 Feedback
Chapter 2. Introduction to C++ and the Standard Library
2.1 History
2.2 New Language Features
2.3 Complexity and the Big-O Notation
Chapter 3. General Concepts
3.1 Namespace
3.2 Header Files
3.3 Error and Exception Handling
3.4 Allocators
Chapter 4. Utilities
4.1 Pairs
4.1.1 Convenience Function
4.1.2 Examples of Pair Usage
4.2 Class
4.3 Numeric Limits
4.4 Auxiliary Functions
4.5 Supplementary Comparison Operators
4.6 Header Files
Chapter 5. The Standard Template Library
5.1 STL Components
5.2 Containers
5.3 Iterators
5.4 Algorithms
5.5 Iterator Adapters
5.6 Manipulating Algorithms
5.7 User-Defined Generic Functions
5.8 Functions as Algorithm Arguments
5.9 Function Objects
5.10 Container Elements
5.11 Errors and Exceptions Inside the STL
5.12 Extending the STL
Chapter 6. STL Containers
6.1 Common Container Abilities and Operations
6.2 Vectors
6.3 Deques
6.4 Lists
6.5 Sets and Multisets
6.6 Maps and Multimaps
6.7 Other STL Containers
6.8 Implementing Reference Semantics
6.9 When to Use which Container
6.10 Container Types and Members in Detail
Chapter 7. STL Iterators
7.1 Header Files for Iterators
7.2 Iterator Categories
7.3 Auxiliary Iterator Functions
7.4 Iterator Adapters
7.5 Iterator Traits
Chapter 8. STL Function Objects
8.1 The Concept of Function Objects
8.2 Predefined Function Objects
8.3 Supplementary Composing Function Objects
Chapter 9. STL Algorithms
9.1 Algorithm Header Files
9.2 Algorithm Overview
9.3 Auxiliary Functions
9.4 The
9.5 Nonmodifying Algorithms
9.6 Modifying Algorithms
9.7 Removing Algorithms
9.8 Mutating Algorithms
9.9 Sorting Algorithms
9.10 Sorted Range Algorithms
9.11 Numeric Algorithms
Chapter 10. Special Containers
10.1 Stacks
10.2 Queues
10.3 Priority Queues
10.4 Bitsets
Chapter 11. Strings
11.1 Motivation
11.2 Description of the String Classes
11.3 String Class in Detail
Chapter 12. Numerics
12.1 Complex Numbers
12.2 Valarrays
12.3 Global Numeric Functions
Chapter 13. Input/Output Using Stream Classes
13.1 Common Background of I/O Streams
13.2 Fundamental Stream Classes and Objects
13.3 Standard Stream Operators << and >>
13.4 State of Streams
13.5 Standard Input/Output Functions
13.6 Manipulators
13.7 Formatting
13.8 Internationalization
13.9 File Access
13.10 Connecting Input and Output Streams
13.11 Stream Classes for Strings
13.12 Input/Output Operators for User-Defined Types
13.13 The Stream Buffer Classes
13.14 Performance Issues
Chapter 14. Internationalization
14.1 Different Character Encodings
14.2 The Concept of Locales
14.3 Locales in Detail
14.4 Facets in Detail
Chapter 15. Allocators
15.1 Using Allocators as an Application Programmer
15.2 Using Allocators as a Library Programmer
15.3 The Default Allocator
15.4 A User-Defined Allocator
15.5 Allocators in Detail
15.6 Utilities for Uninitialized Memory in Detail
Internet Resources
Bibliography
Download
Another C++ books
Another Programming Language books
No comments:
Post a Comment