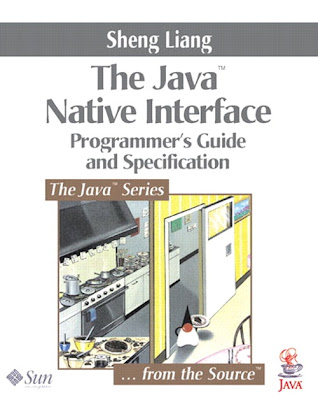
Contents
Preface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xiii
Part One: Introduction and Tutorial
1 Introduction. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.1 The Java Platform and Host Environment . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.2 Role of the JNI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.3 Implications of Using the JNI. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.4 When to Use the JNI. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.5 Evolution of the JNI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
1.6 Example Programs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2 Getting Started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.1 Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.2 Declare the Native Method. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
2.3 Compile the HelloWorld Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2.4 Create the Native Method Header File . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2.5 Write the Native Method Implementation . . . . . . . . . . . . . . . . . . . . . . . . . 15
2.6 Compile the C Source and Create a Native Library . . . . . . . . . . . . . . . . . . 15
2.7 Run the Program. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
Part Two: Programmer’s Guide
3 Basic Types, Strings, and Arrays. . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.1 A Simple Native Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.1.1 C Prototype for Implementing the Native Method. . . . . . . . . . . . 22
3.1.2 Native Method Arguments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
3.1.3 Mapping of Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
3.2 Accessing Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
3.2.1 Converting to Native Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
3.2.2 Freeing Native String Resources . . . . . . . . . . . . . . . . . . . . . . . . . 25
3.2.3 Constructing New Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
3.2.4 Other JNI String Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
3.2.5 New JNI String Functions in Java 2 SDK Release 1.2 . . . . . . . . 27
3.2.6 Summary of JNI String Functions. . . . . . . . . . . . . . . . . . . . . . . . 29
3.2.7 Choosing among the String Functions . . . . . . . . . . . . . . . . . . . . 31
3.3 Accessing Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
3.3.1 Accessing Arrays in C. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
3.3.2 Accessing Arrays of Primitive Types . . . . . . . . . . . . . . . . . . . . . 34
3.3.3 Summary of JNI Primitive Array Functions . . . . . . . . . . . . . . . . 35
3.3.4 Choosing among the Primitive Array Functions . . . . . . . . . . . . . 36
3.3.5 Accessing Arrays of Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
4 Fields and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.1 Accessing Fields . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.1.1 Procedure for Accessing an Instance Field . . . . . . . . . . . . . . . . . 43
4.1.2 Field Descriptors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.1.3 Accessing Static Fields . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.2 Calling Methods. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
4.2.1 Calling Instance Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
4.2.2 Forming the Method Descriptor . . . . . . . . . . . . . . . . . . . . . . . . . 48
4.2.3 Calling Static Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
4.2.4 Calling Instance Methods of a Superclass. . . . . . . . . . . . . . . . . . 51
4.3 Invoking Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
4.4 Caching Field and Method IDs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
4.4.1 Caching at the Point of Use. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
4.4.2 Caching in the Defining Class’s Initializer . . . . . . . . . . . . . . . . . 56
4.4.3 Comparison between the Two Approaches to Caching IDs . . . . 57
4.5 Performance of JNI Field and Method Operations . . . . . . . . . . . . . . . . . . 58
5 Local and Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.1 Local and Global References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.1.1 Local References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
5.1.2 Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
5.1.3 Weak Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
5.1.4 Comparing References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
5.2 Freeing References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
5.2.1 Freeing Local References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67
5.2.2 Managing Local References in Java 2 SDK Release 1.2 . . . . . . 68
5.2.3 Freeing Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
5.3 Rules for Managing References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 70
6 Exceptions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
6.1 Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
6.1.1 Caching and Throwing Exceptions in Native Code . . . . . . . . . . 73
6.1.2 A Utility Function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75
6.2 Proper Exception Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
6.2.1 Checking for Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
6.2.2 Handling Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 78
6.2.3 Exceptions in Utility Functions. . . . . . . . . . . . . . . . . . . . . . . . . . 79
7 The Invocation Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
7.1 Creating the Java Virtual Machine . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
7.2 Linking Native Applications with the Java Virtual Machine . . . . . . . . . . . 86
7.2.1 Linking with a Known Java Virtual Machine . . . . . . . . . . . . . . . 86
7.2.2 Linking with Unknown Java Virtual Machines . . . . . . . . . . . . . . 87
7.3 Attaching Native Threads . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89
8 Additional JNI Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1 JNI and Threads . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1.1 Constraints . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1.2 Monitor Entry and Exit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 94
8.1.3 Monitor Wait and Notify . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 95
8.1.4 Obtaining a JNIEnv Pointer in Arbitrary Contexts . . . . . . . . . . . 96
8.1.5 Matching the Thread Models . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97
8.2 Writing Internationalized Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 99
8.2.1 Creating jstrings from Native Strings . . . . . . . . . . . . . . . . . . . 99
8.2.2 Translating jstrings to Native Strings . . . . . . . . . . . . . . . . . . 100
8.3 Registering Native Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 101
8.4 Load and Unload Handlers. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
8.4.1 The JNI_OnLoad Handler. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
8.4.2 The JNI_OnUnload Handler. . . . . . . . . . . . . . . . . . . . . . . . . . . 104
8.5 Reflection Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105
8.6 JNI Programming in C++ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106
9 Leveraging Existing Native Libraries . . . . . . . . . . . . . . . . . . . . . 109
9.1 One-to-One Mapping . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 109
9.2 Shared Stubs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113
9.3 One-to-One Mapping versus Shared Stubs . . . . . . . . . . . . . . . . . . . . . . . 116
9.4 Implementation of Shared Stubs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 116
9.4.1 The CPointer Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117
9.4.2 The CMalloc Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117
9.4.3 The CFunction Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118
9.5 Peer Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
9.5.1 Peer Classes in the Java Platform. . . . . . . . . . . . . . . . . . . . . . . . 124
9.5.2 Freeing Native Data Structures . . . . . . . . . . . . . . . . . . . . . . . . . 125
9.5.3 Backpointers to Peer Instances . . . . . . . . . . . . . . . . . . . . . . . . . 127
10 Traps and Pitfalls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
10.1 Error Checking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
10.2 Passing Invalid Arguments to JNI Functions . . . . . . . . . . . . . . . . . . . . . . 131
10.3 Confusing jclass with jobject . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
10.4 Truncating jboolean Arguments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
10.5 Boundaries between Java Application and Native Code . . . . . . . . . . . . . 133
10.6 Confusing IDs with References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134
10.7 Caching Field and Method IDs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135
10.8 Terminating Unicode Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137
10.9 Violating Access Control Rules. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137
10.10 Disregarding Internationalization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 138
10.11 Retaining Virtual Machine Resources . . . . . . . . . . . . . . . . . . . . . . . . . . . 139
10.12 Excessive Local Reference Creation . . . . . . . . . . . . . . . . . . . . . . . . . . . . 140
10.13 Using Invalid Local References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
10.14 Using the JNIEnv across Threads . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
10.15 Mismatched Thread Models . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
Part Three: Specification
11 Overview of the JNI Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145
11.1 Design Goals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145
11.2 Loading Native Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 146
11.2.1 Class Loaders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 146
11.2.2 Class Loaders and Native Libraries. . . . . . . . . . . . . . . . . . . . . . 147
11.2.3 Locating Native Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 148
11.2.4 A Type Safety Restriction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 150
11.2.5 Unloading Native Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151
11.3 Linking Native Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151
11.4 Calling Conventions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153
11.5 The JNIEnv Interface Pointer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153
11.5.1 Organization of the JNIEnv Interface Pointer . . . . . . . . . . . . . 153
11.5.2 Benefits of an Interface Pointer . . . . . . . . . . . . . . . . . . . . . . . . . 155
11.6 Passing Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 155
11.6.1 Global and Local References . . . . . . . . . . . . . . . . . . . . . . . . . . 156
11.6.2 Implementing Local References . . . . . . . . . . . . . . . . . . . . . . . . 157
11.6.3 Weak Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
11.7 Accessing Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
11.7.1 Accessing Primitive Arrays. . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
11.7.2 Fields and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 160
11.8 Errors and Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161
11.8.1 No Checking for Programming Errors . . . . . . . . . . . . . . . . . . . 161
11.8.2 Java Virtual Machine Exceptions . . . . . . . . . . . . . . . . . . . . . . . 162
11.8.3 Asynchronous Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 163
12 JNI Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
12.1 Primitive and Reference Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
12.1.1 Primitive Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
12.1.2 Reference Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 166
12.1.3 The jvalue Type . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167
12.2 Field and Method IDs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168
12.3 String Formats . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168
12.3.1 UTF-8 Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168
12.3.2 Class Descriptors. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169
12.3.3 Field Descriptors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169
12.3.4 Method Descriptors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
12.4 Constants . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
13 JNI Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 173
13.1 Summary of the JNI Functions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 173
13.1.1 Directly-Exported Invocation Interface Functions. . . . . . . . . . . 173
13.1.2 The JavaVM Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
13.1.3 Functions Defined in Native Libraries . . . . . . . . . . . . . . . . . . . . 175
13.1.4 The JNIEnv Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 175
13.2 Specification of JNI Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 180
Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 293
Download this book click here
Related books click here
No comments:
Post a Comment